美团2024年秋招第六场笔试-技术
选择
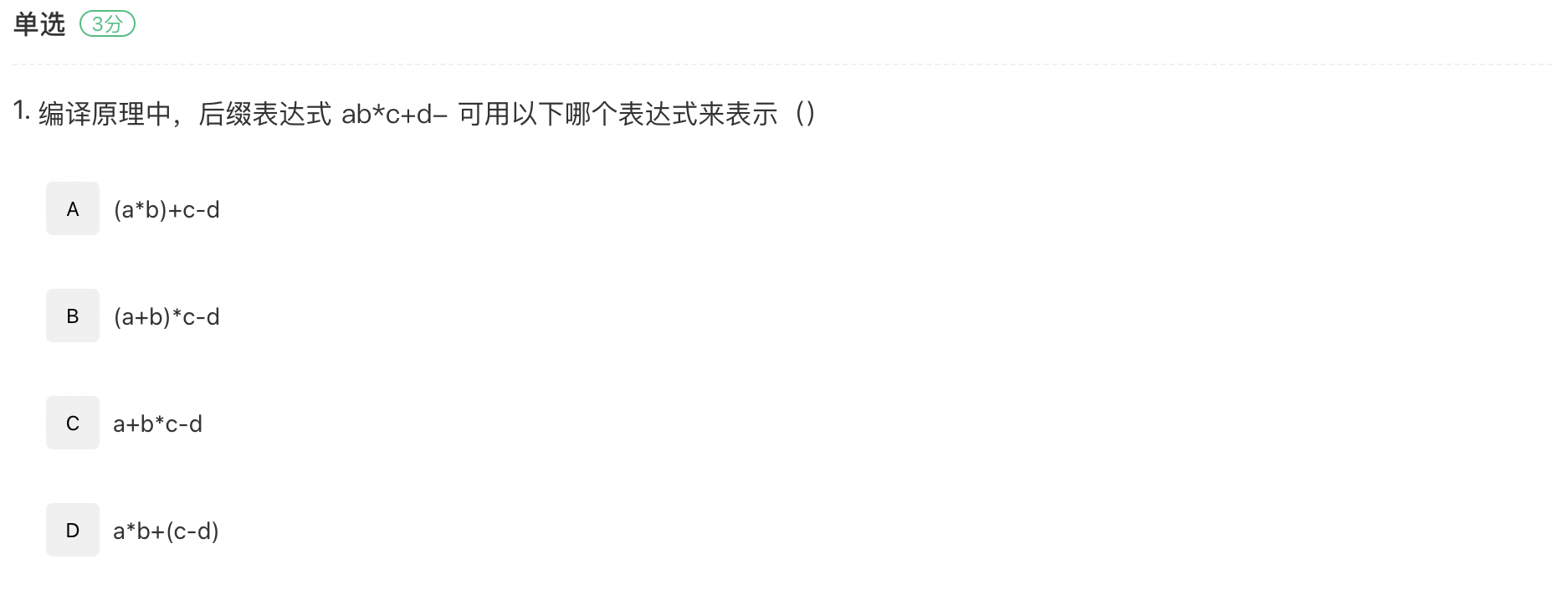
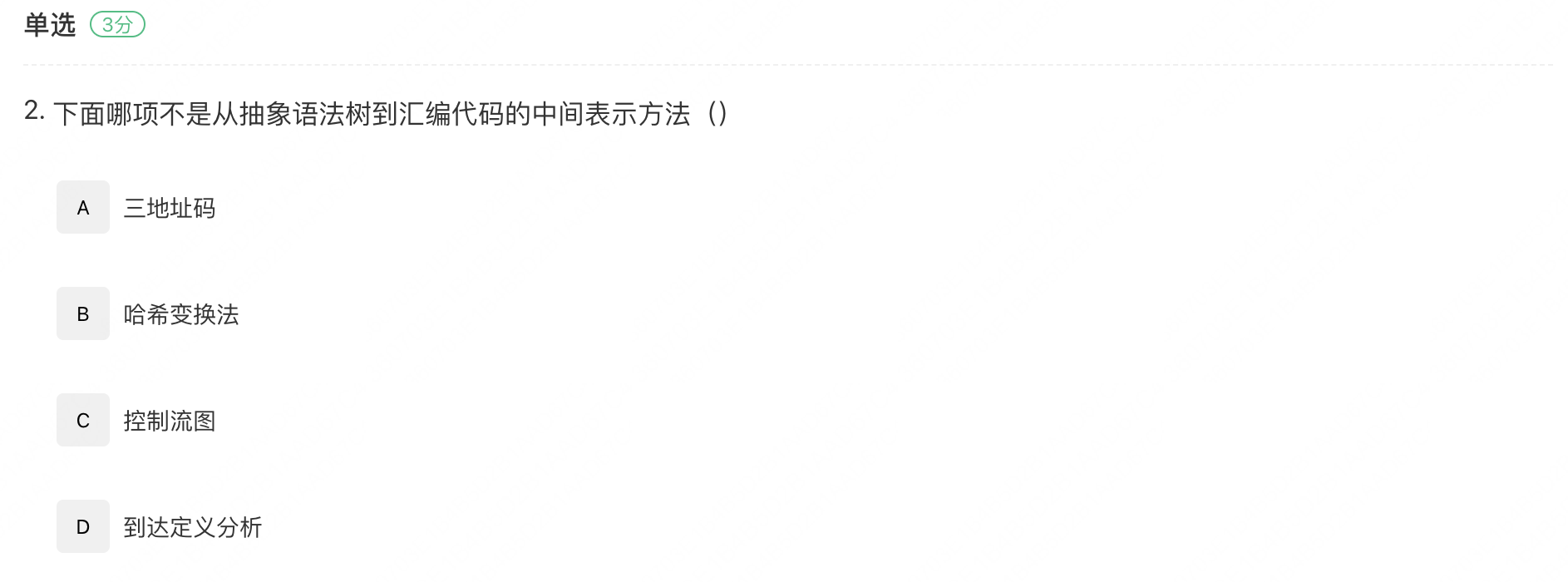
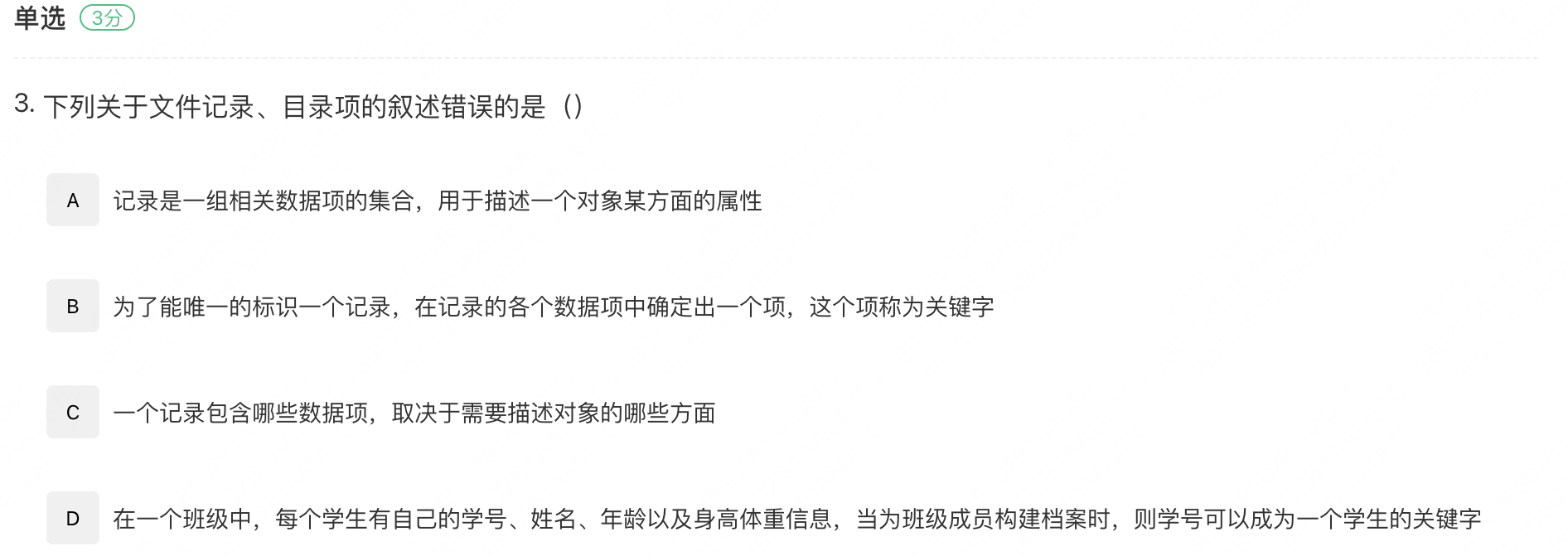
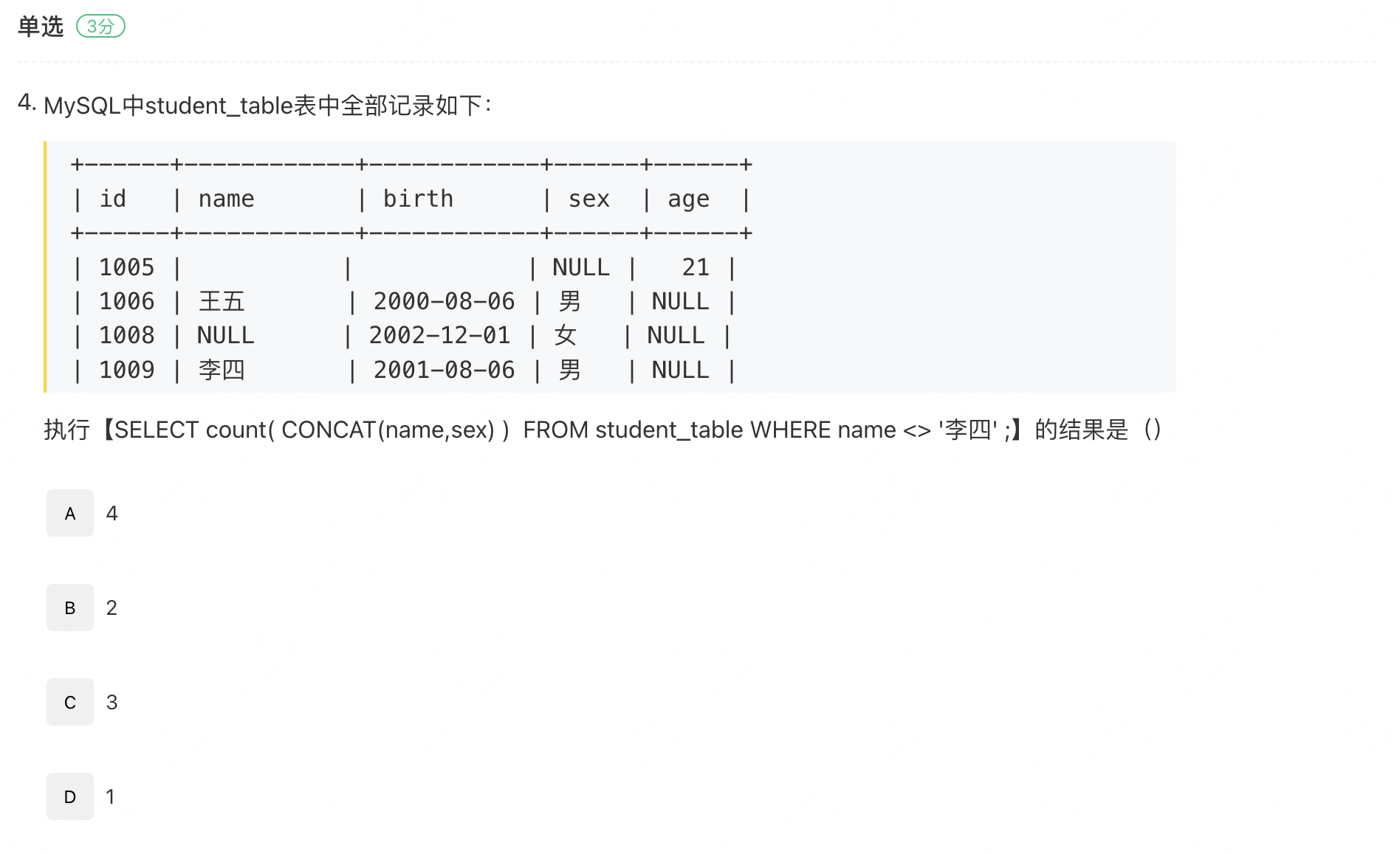
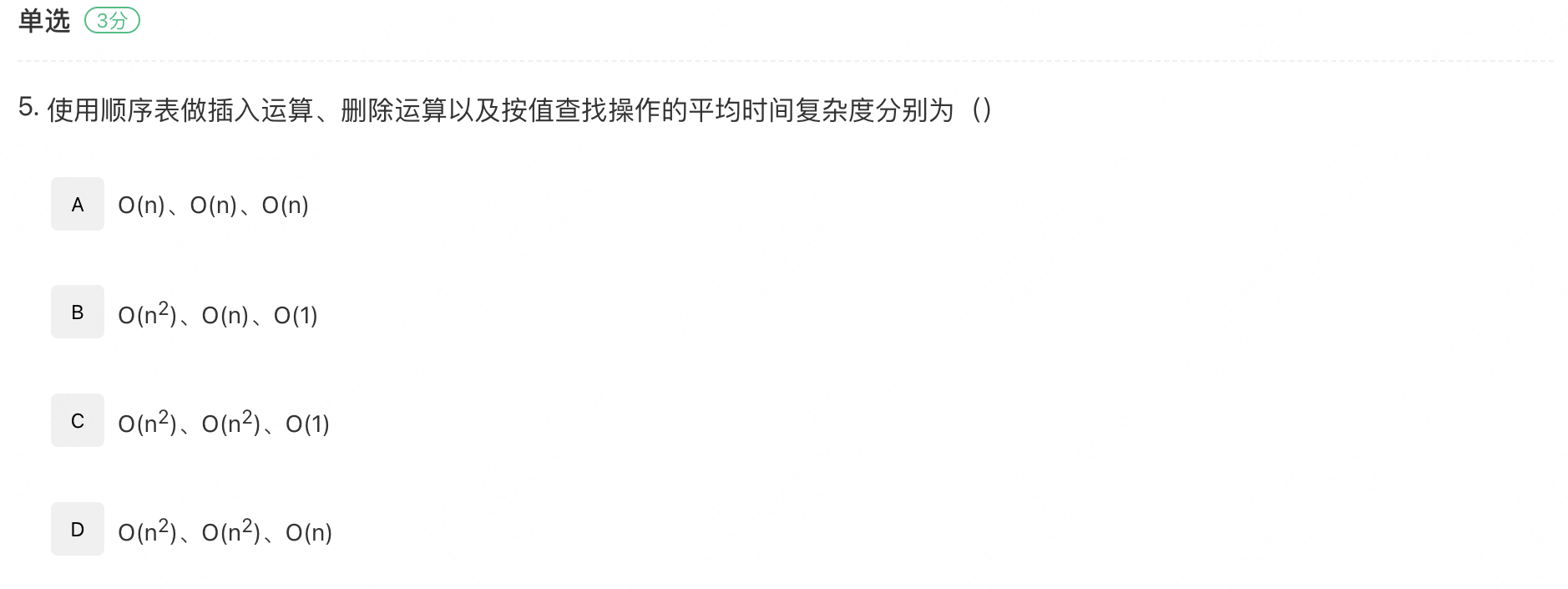
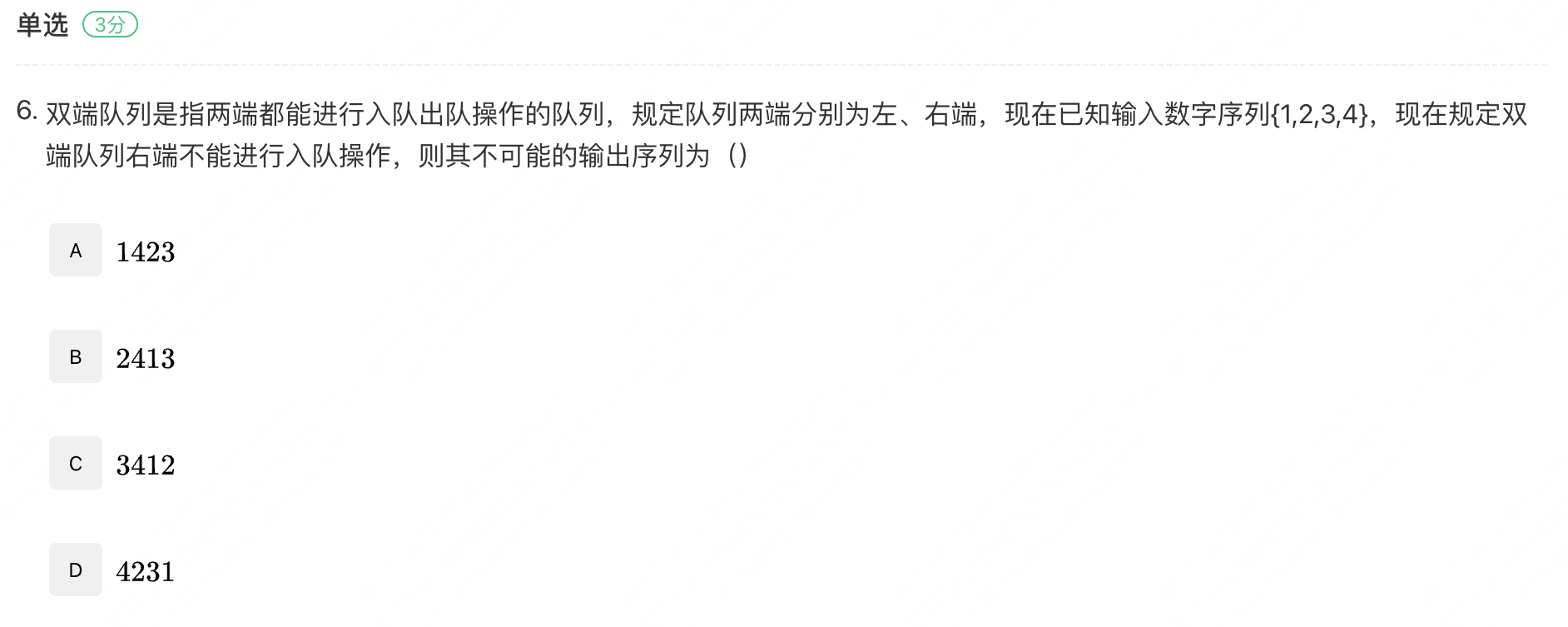
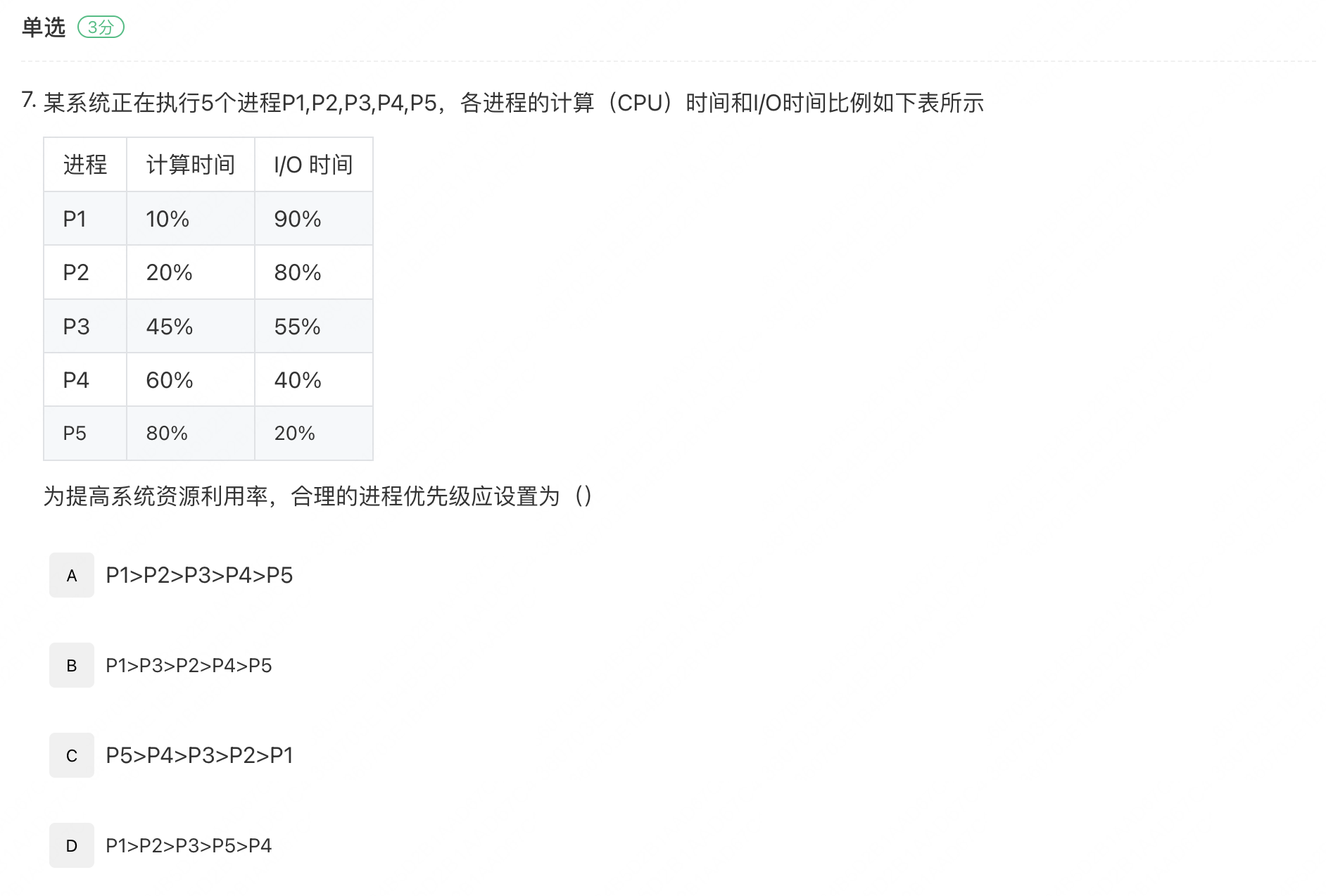
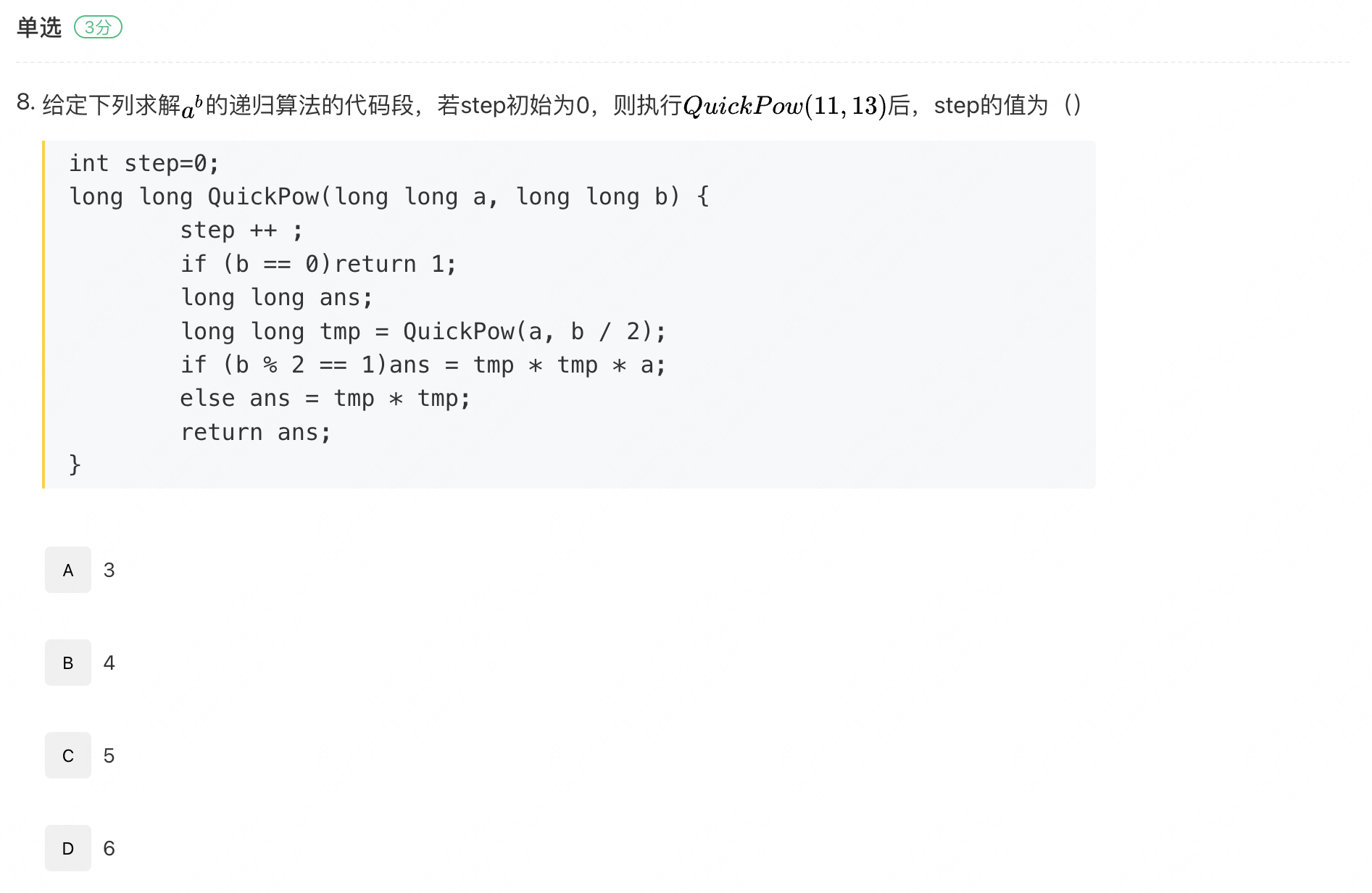
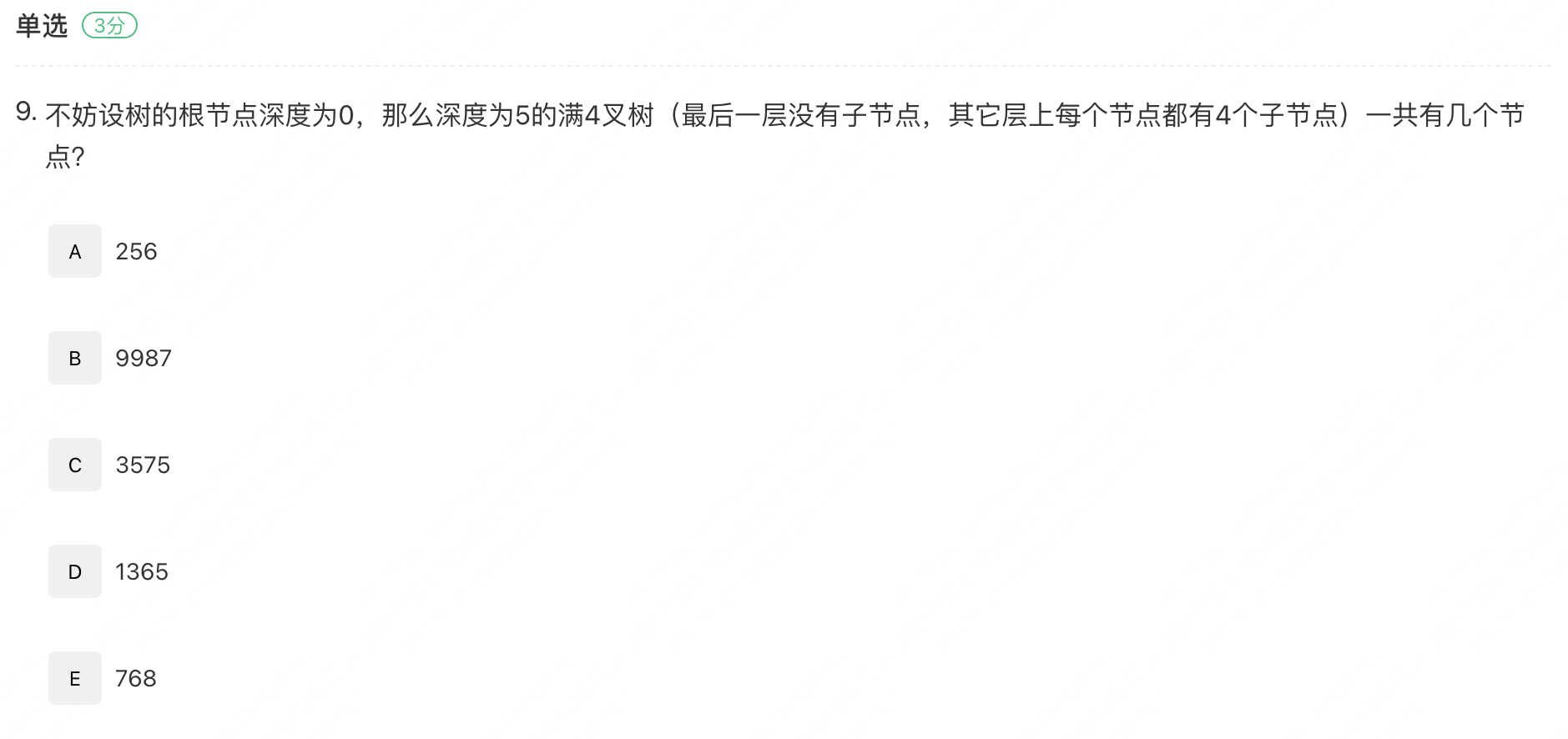
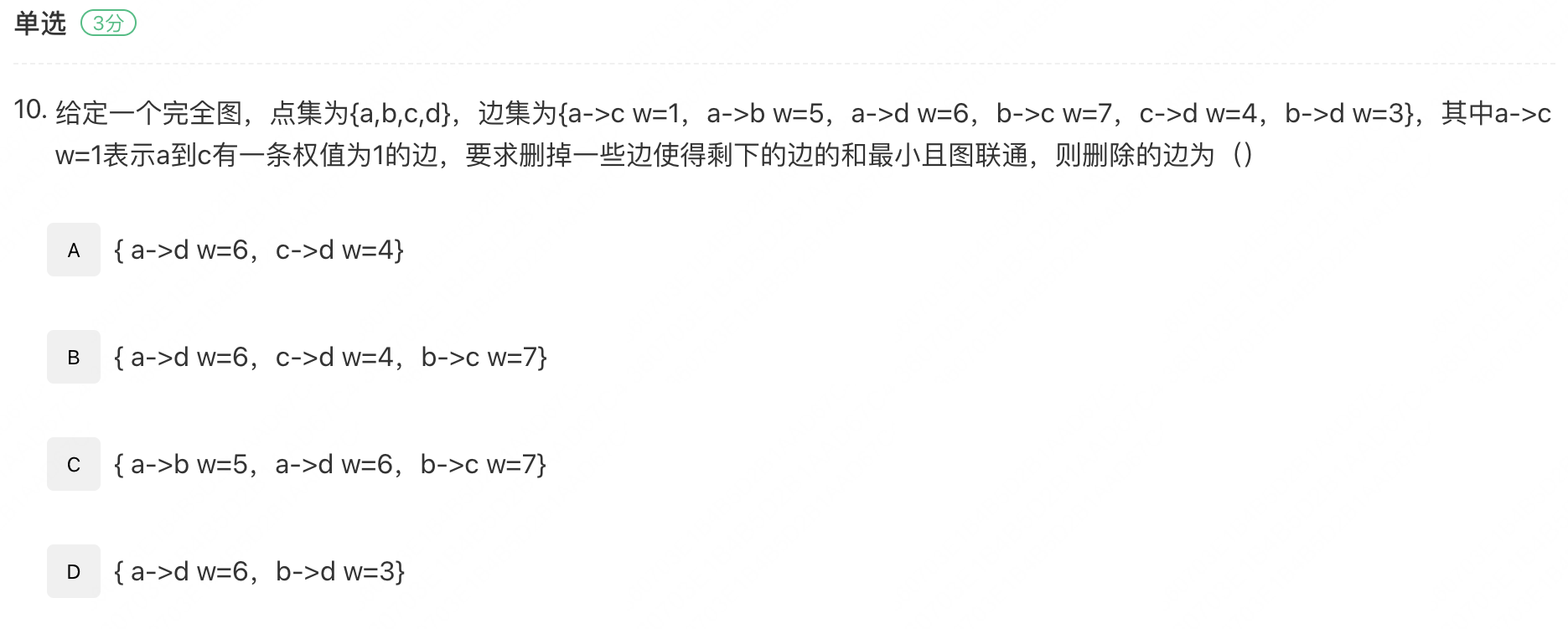
编程
1. 捉迷藏
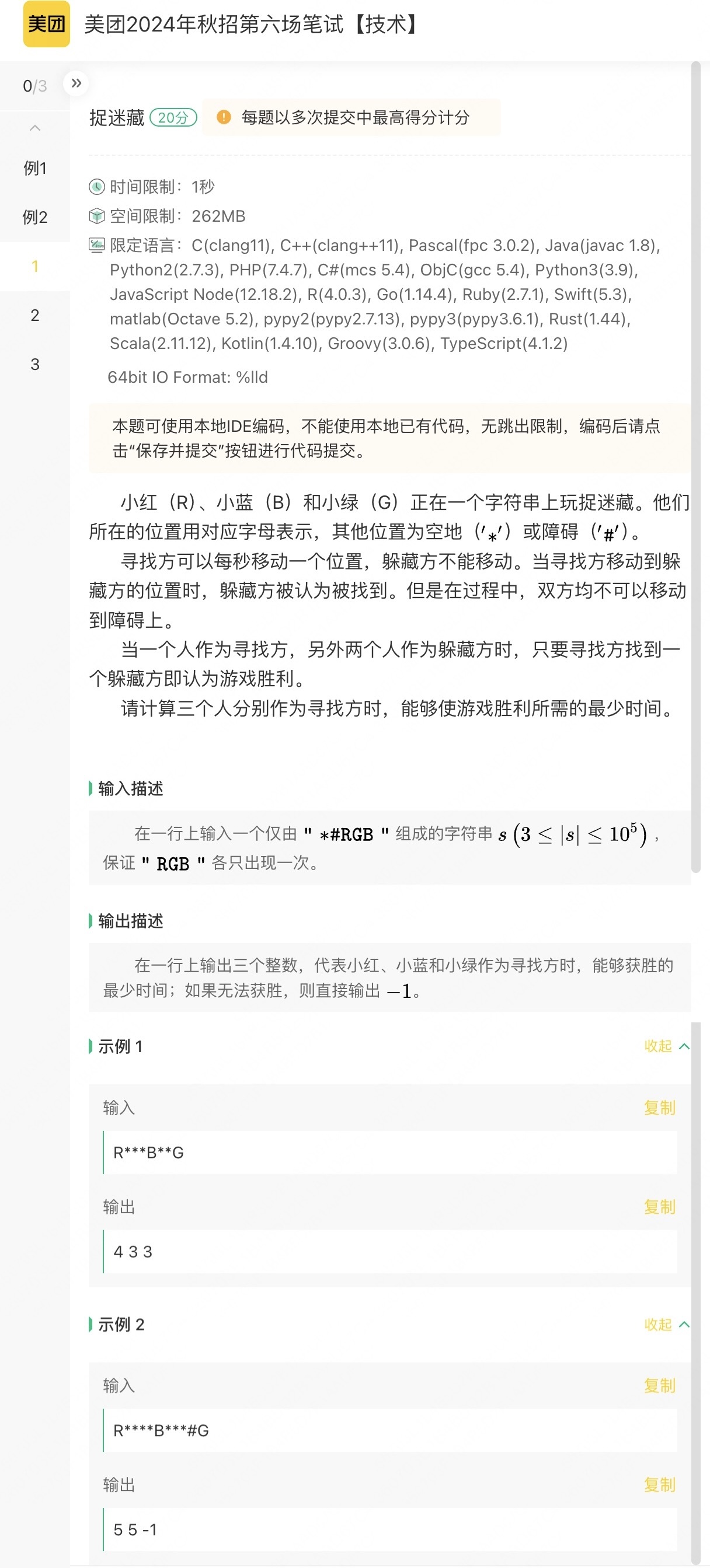
cpp
// Description: 美团2024年秋招第六场笔试-技术-编程第一题
// Accept: 100%
// Date: 2024/09/14
#include <iostream>
#include <string>
#include <vector>
#include <cmath>
using namespace std;
int found(int founder, int hider, const vector<int>& sharps) {
if (founder < hider) { // founder在左边
for (int sharp : sharps) {
if (sharp > founder && sharp < hider) {
return -1;
}
}
} else {
for (int sharp : sharps) {
if (sharp > hider && sharp < founder) {
return -1;
}
}
}
return fabs(hider - founder);
}
int found(int founder, int hider1, int hider2, const vector<int>& sharps) {
int distenceToCatchHider1 = found(founder, hider1, sharps);
int distenceToCatchHider2 = found(founder, hider2, sharps);
if (distenceToCatchHider1 > 0 && distenceToCatchHider2 > 0) {
return min(distenceToCatchHider1, distenceToCatchHider2);
} else if (distenceToCatchHider1 == -1) {
return distenceToCatchHider2;
} else if (distenceToCatchHider2 == -1) {
return distenceToCatchHider1;
}
return -1;
}
int main() {
string s;
while (cin >> s) { // 注意 while 处理多个 case
vector<int> sharps;
int R, G, B;
for (int i = 0; i < s.size(); ++i) {
if (s[i] == '#') {
sharps.emplace_back(i);
} else if (s[i] == 'R') {
R = i;
} else if (s[i] == 'G') {
G = i;
} else if (s[i] == 'B') {
B = i;
}
}
cout << found(R, G, B, sharps) << " " << found(B, G, R,
sharps) << " " << found(G, R, B, sharps) << endl;
}
}
// 64 位输出请用 printf("%lld")
2. 小美的砖块
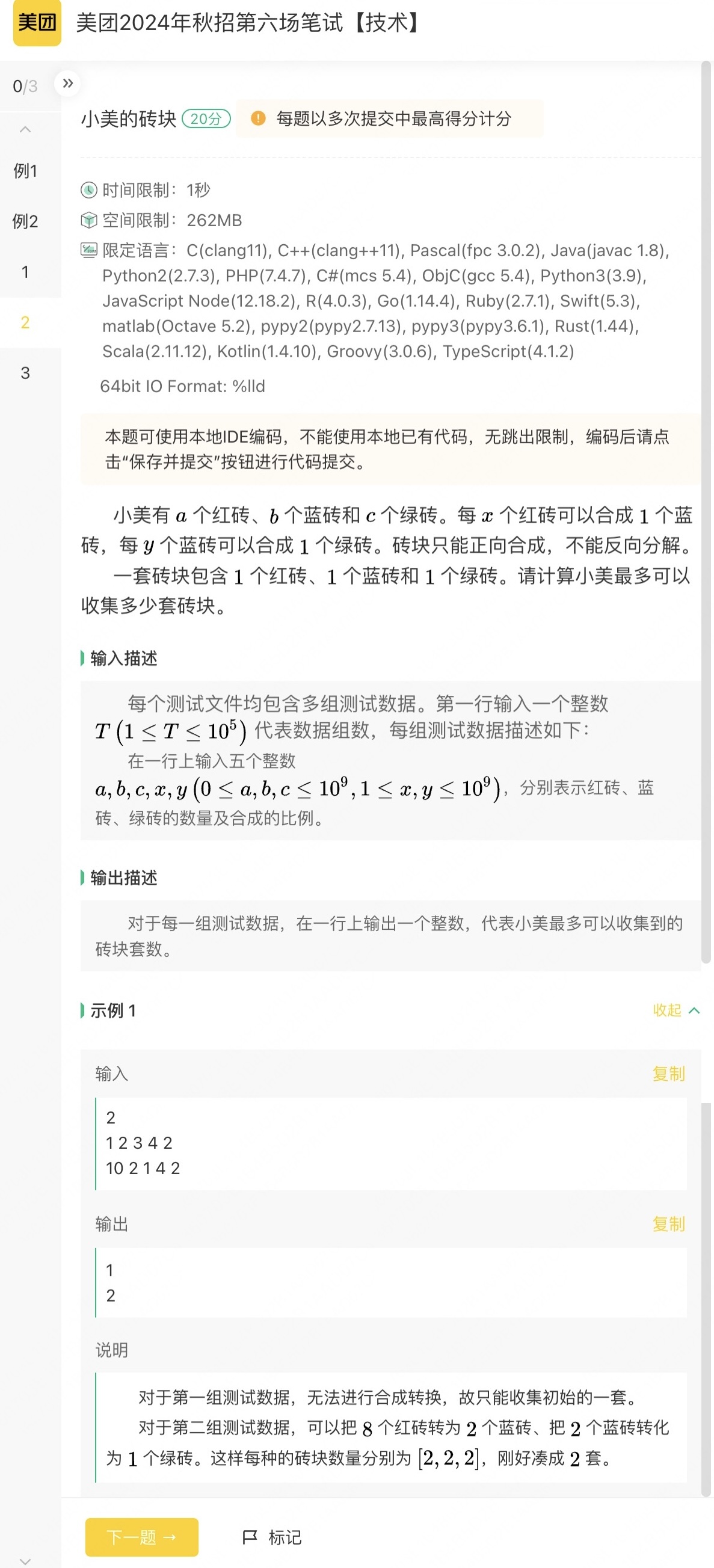
cpp
// Description: 美团2024年秋招第六场笔试-技术-编程第二题
// Accept: 100%
// Date: 2024/09/14
#include <iostream>
#include <algorithm>
using namespace std;
// 做 n 个蓝砖
bool makeNBlue(int& a, int& b, int x, int n) {
if (a < x * n) {
return false; // 红砖不够,无法制作 n 个蓝砖
}
a -= x * n; // 消耗红砖制作蓝砖
b += n; // 增加蓝砖数量
return true;
}
// 做一个绿砖
bool makeGreen(int& a, int& b, int& c, int x, int y) {
if (b < y) {
// 如果蓝砖不够,先制作缺少的蓝砖
int blue_needed = y - b;
if (!makeNBlue(a, b, x, blue_needed)) {
return false; // 如果无法制作足够蓝砖,返回 false
}
}
// 有足够的蓝砖,制作一个绿砖
b -= y;
c += 1;
return true;
}
// 做一套
bool makeSuit(int& a, int& b, int& c, int& res) {
if (a > 0 && b > 0 && c > 0) {
a -= 1;
b -= 1;
c -= 1;
res += 1;
return true;
}
return false;
}
int main() {
int T;
cin >> T;
while (T-- > 0) {
int a, b, c, x, y;
cin >> a >> b >> c >> x >> y;
int res = 0;
// 优先使用已有的红、蓝、绿砖制作套装
while (makeSuit(a, b, c, res)) {}
// 继续尝试制作更多的绿砖和套装
while (true) {
if (c == 0) {
// 如果没有绿砖,尝试制作绿砖
if (!makeGreen(a, b, c, x, y)) {
break; // 无法再制作绿砖,跳出循环
}
}
if (b == 0) {
// 如果没有蓝砖,尝试制作蓝砖
if (!makeNBlue(a, b, x, 1)) {
break; // 无法再制作蓝砖,跳出循环
}
}
// 尝试制作套装
if (!makeSuit(a, b, c, res)) {
break; // 无法再制作套装,跳出循环
}
}
cout << res << endl; // 输出最多可以制作的套装数量
}
return 0;
}
3. 小美的图上游戏
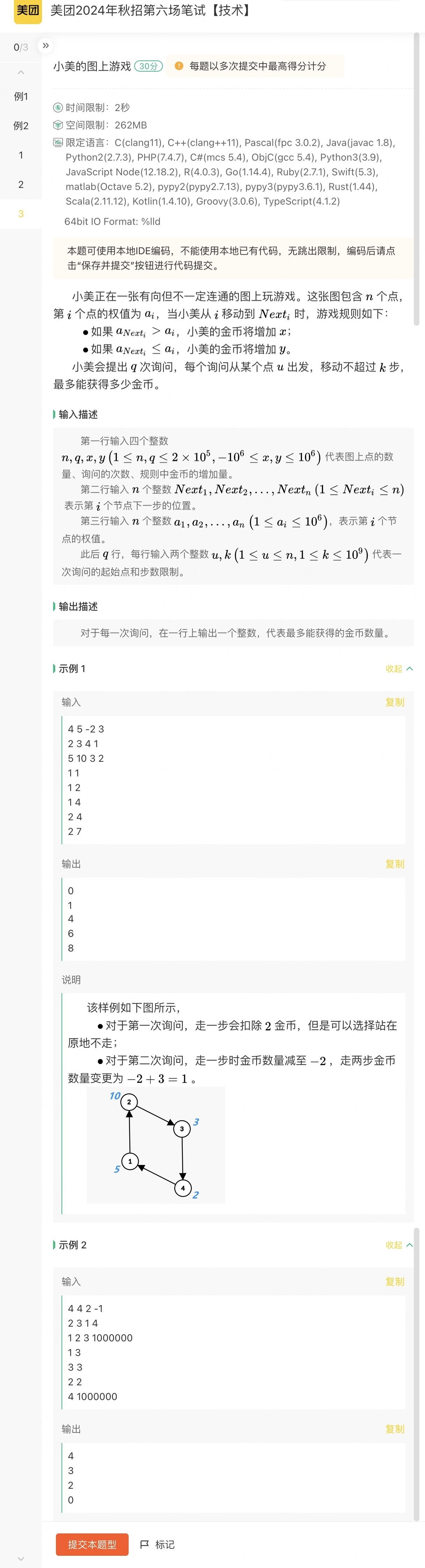