oppo-2025届秋招-移动端B卷-20240914
oppo-2025届秋招-移动端B卷-20240914
时间:2024-09-14 14:15
选择
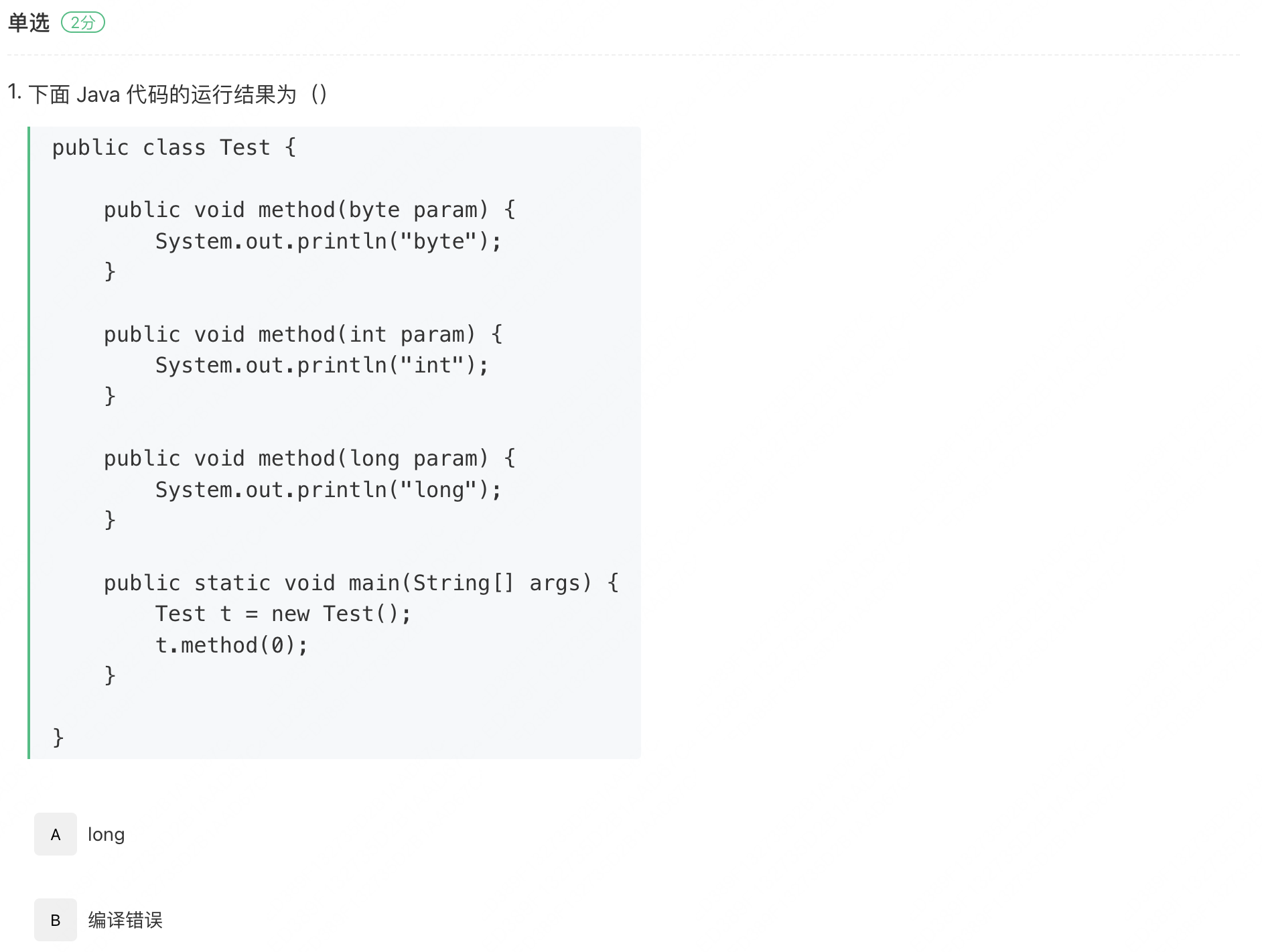

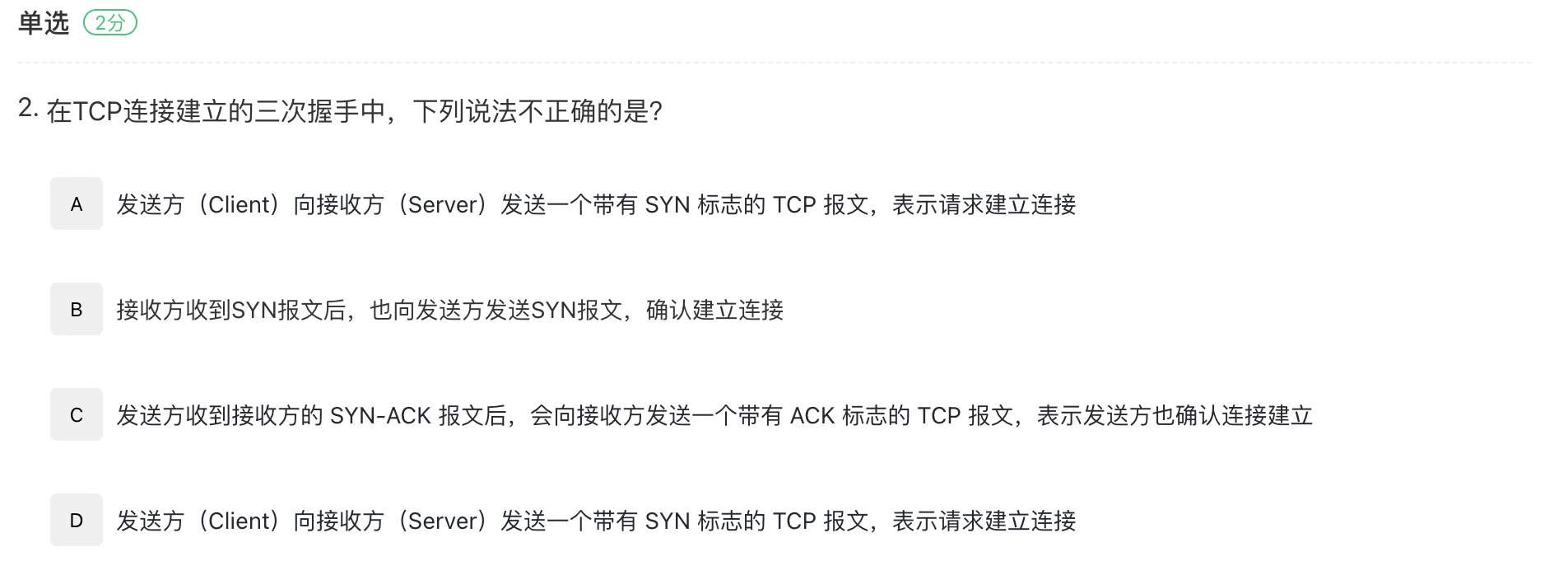
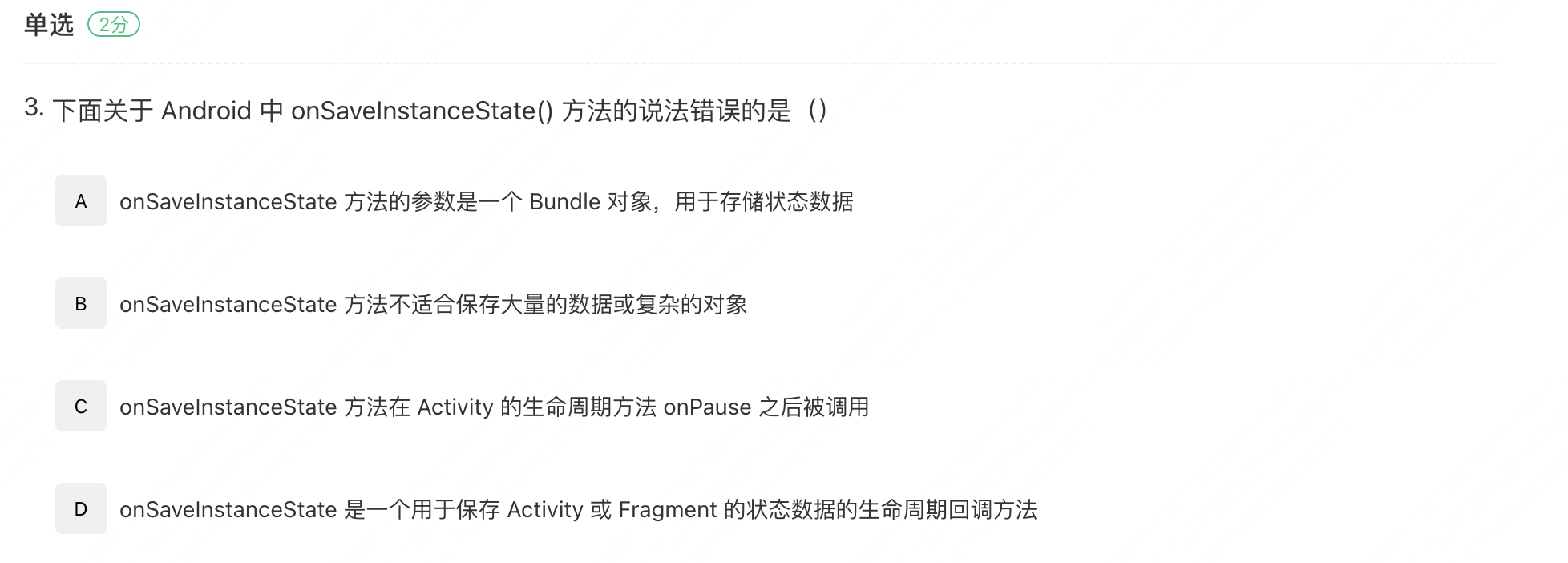
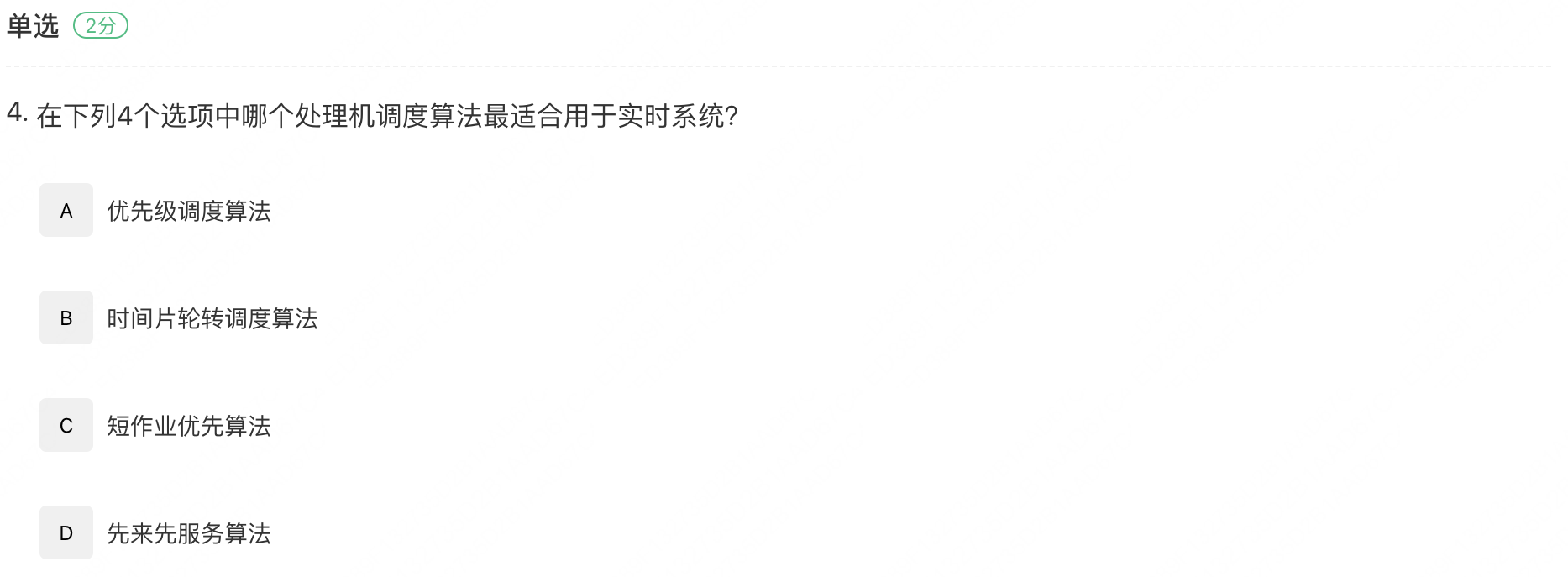
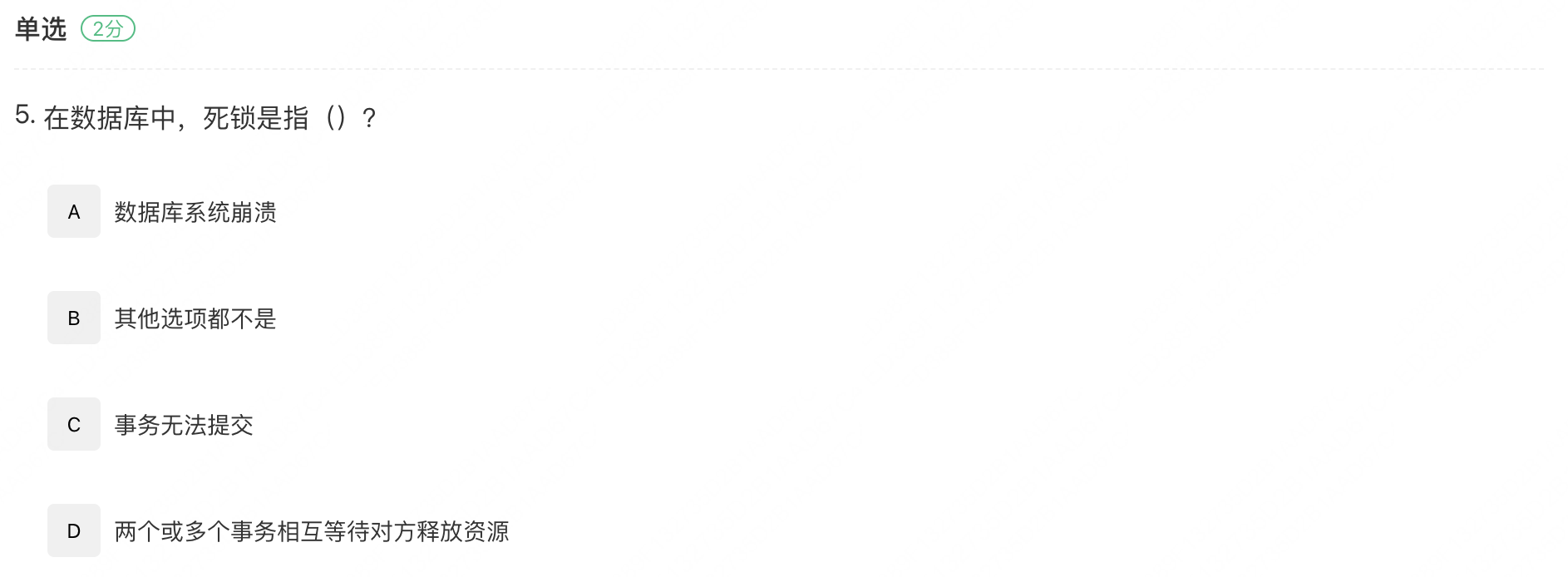
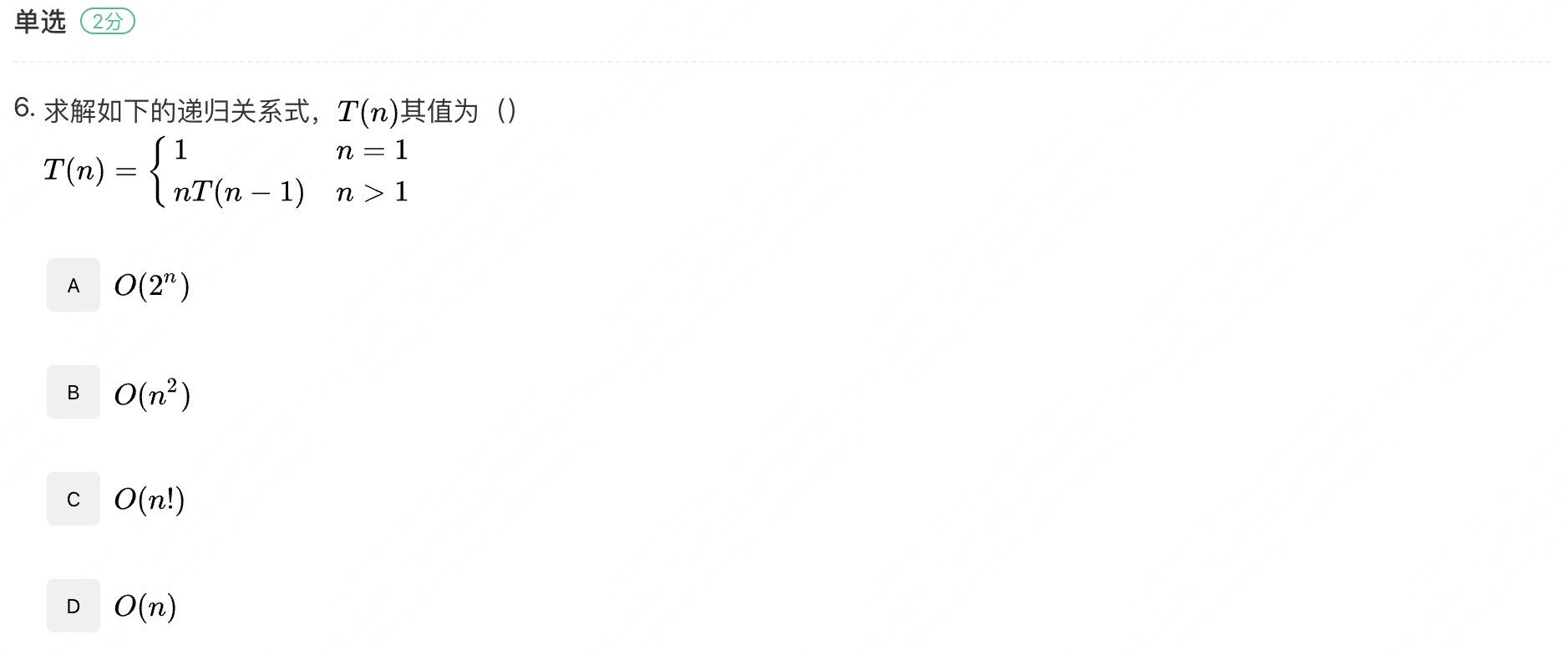
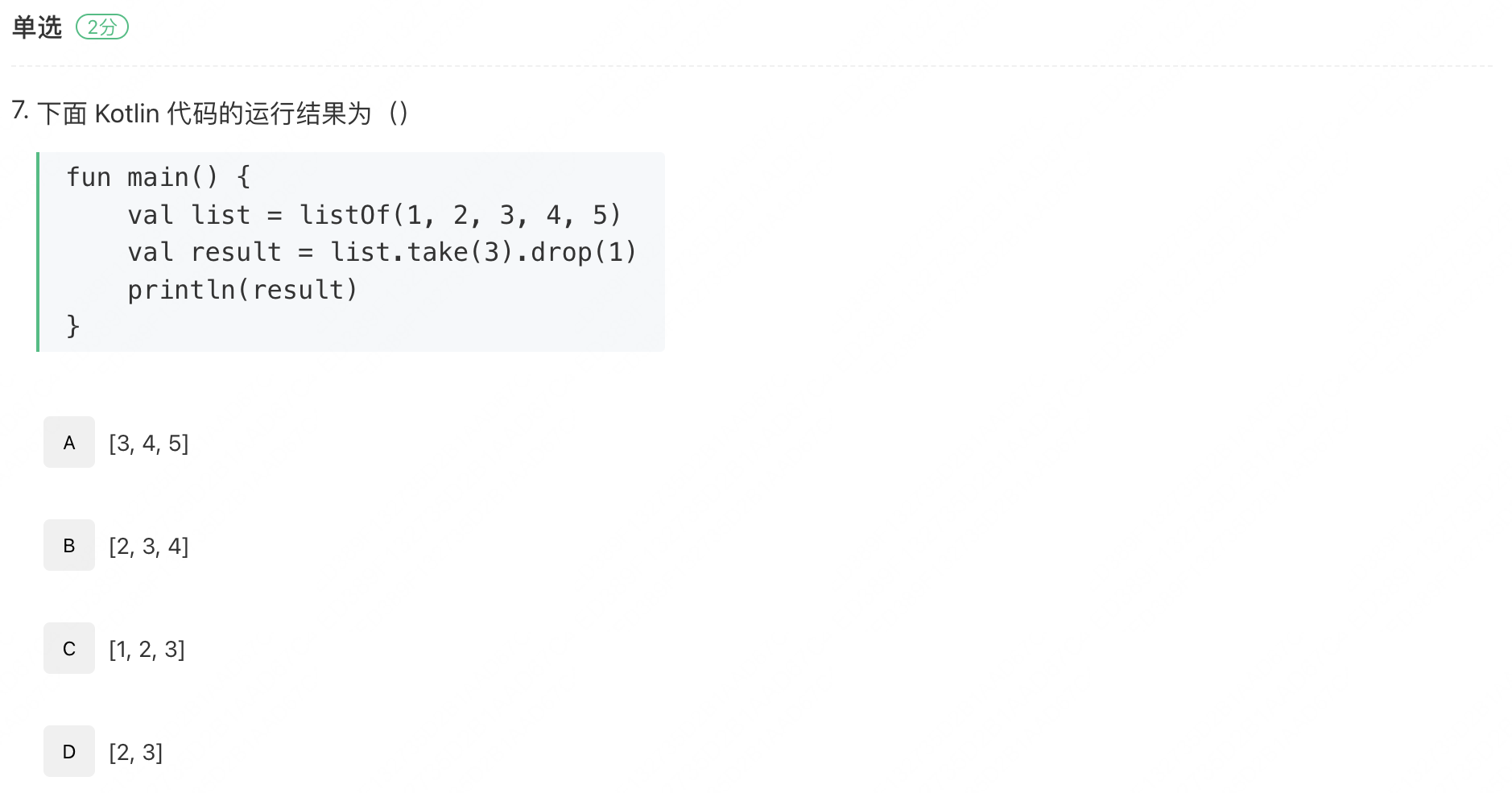
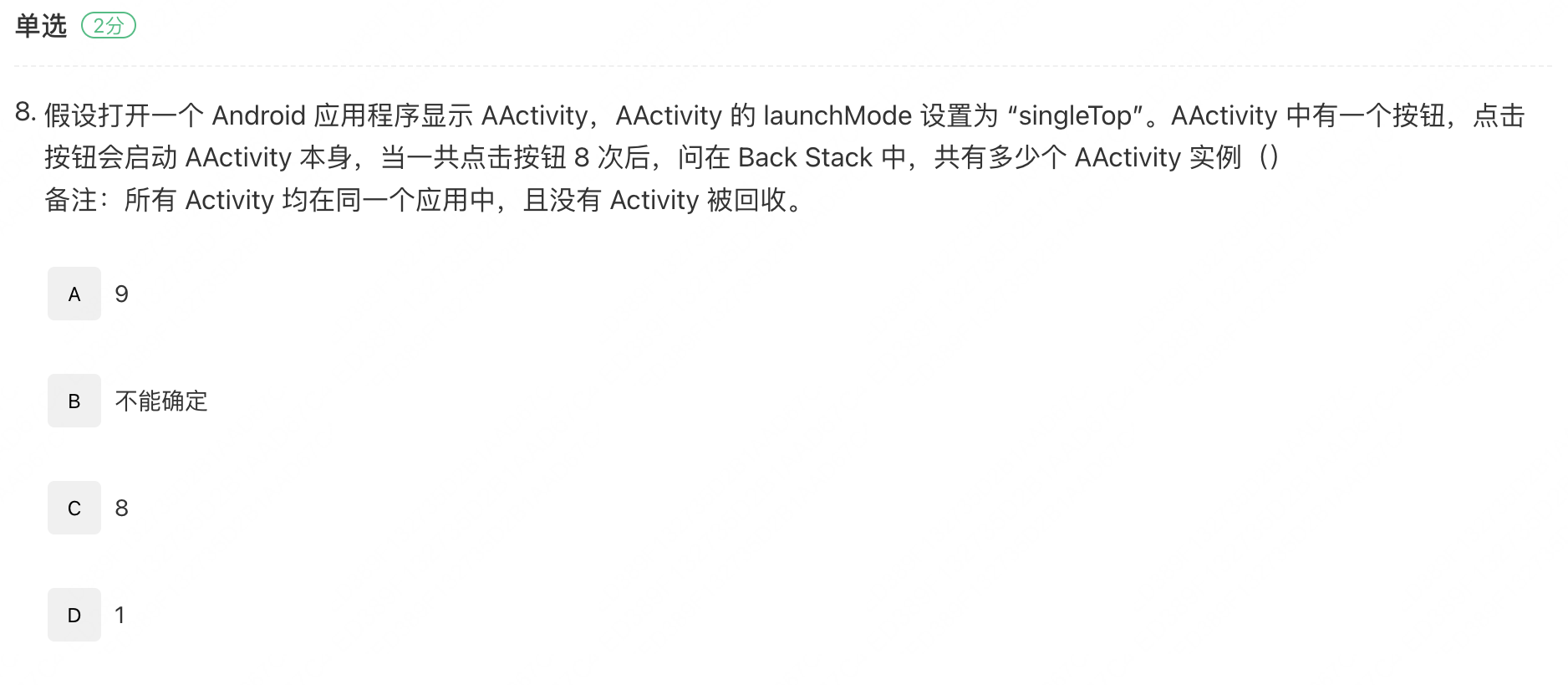
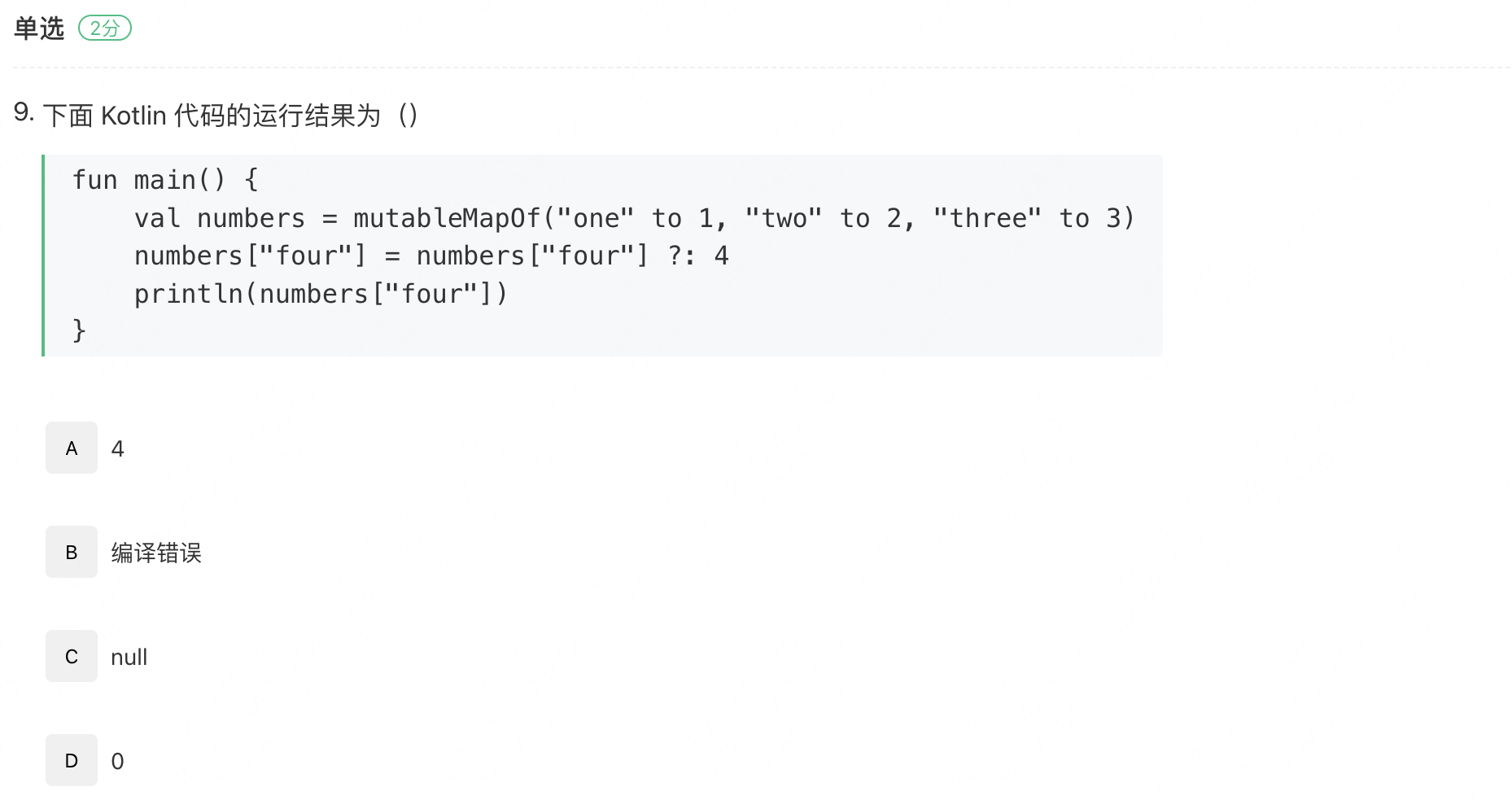
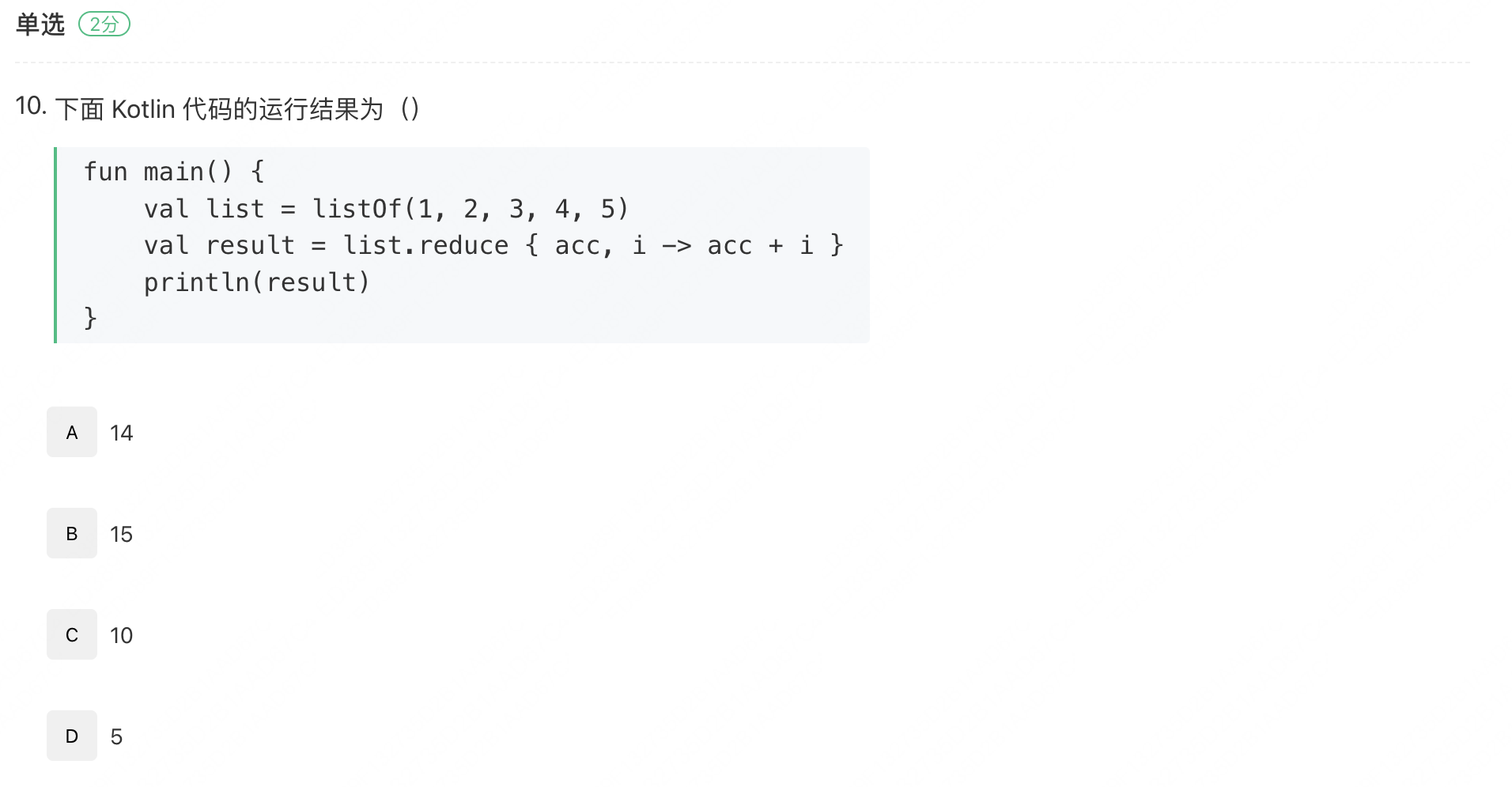
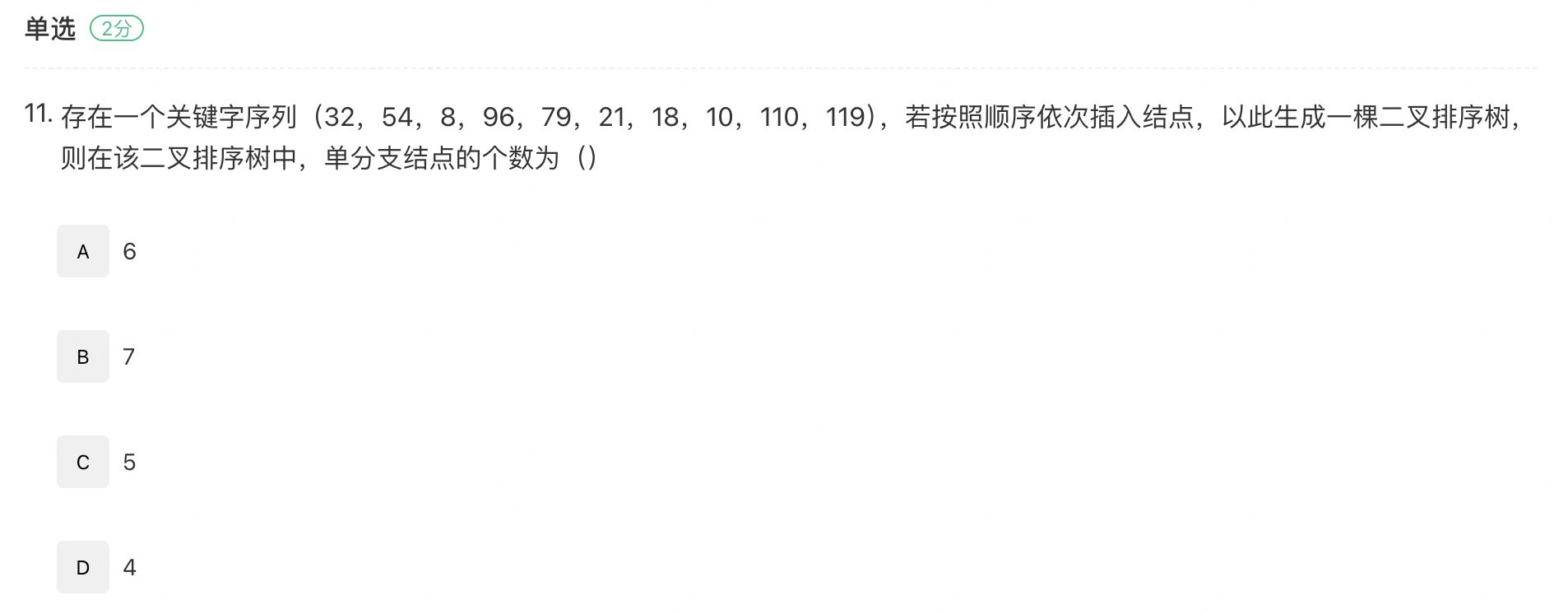
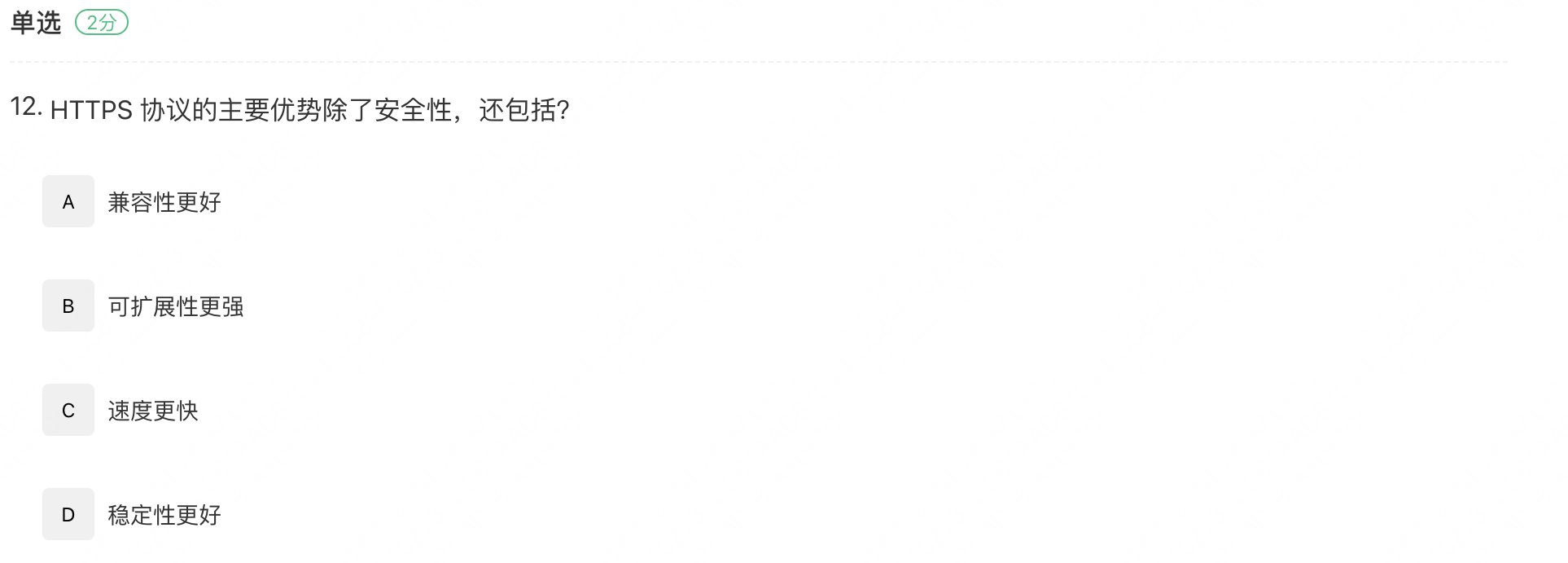
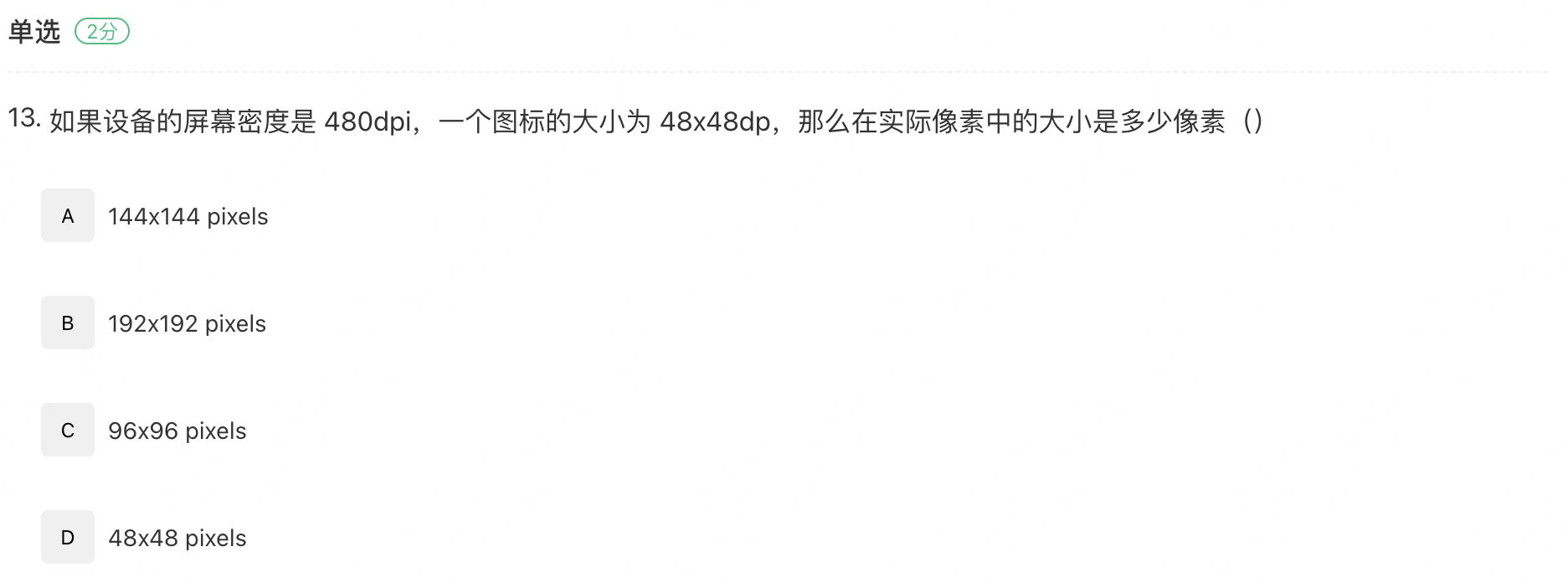
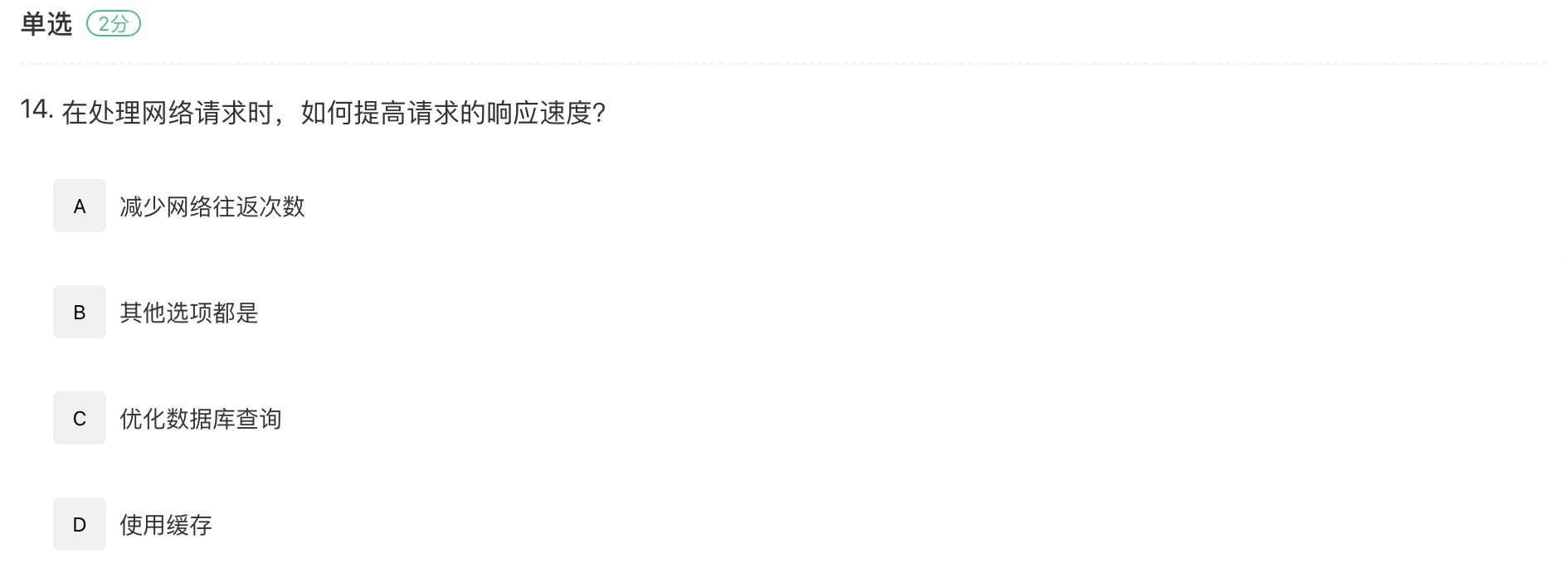
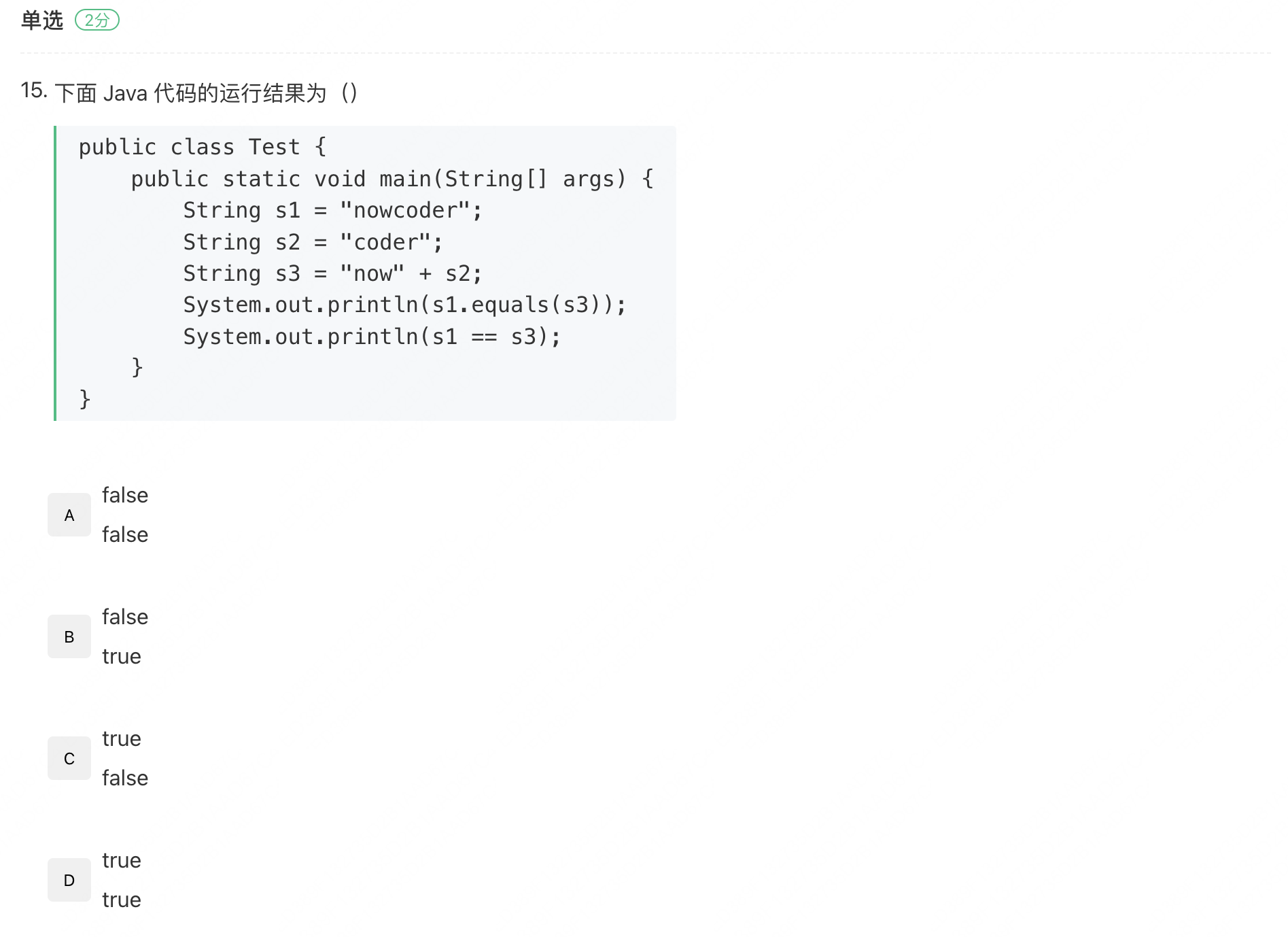
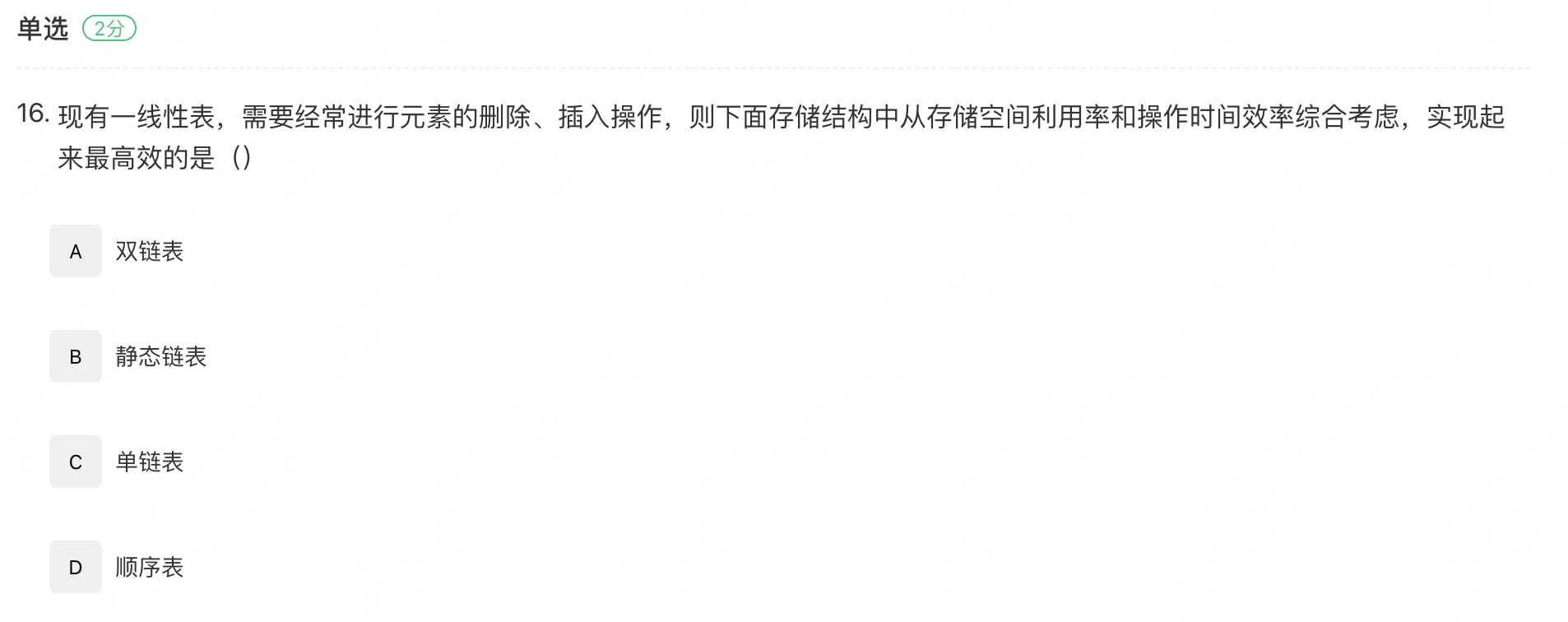
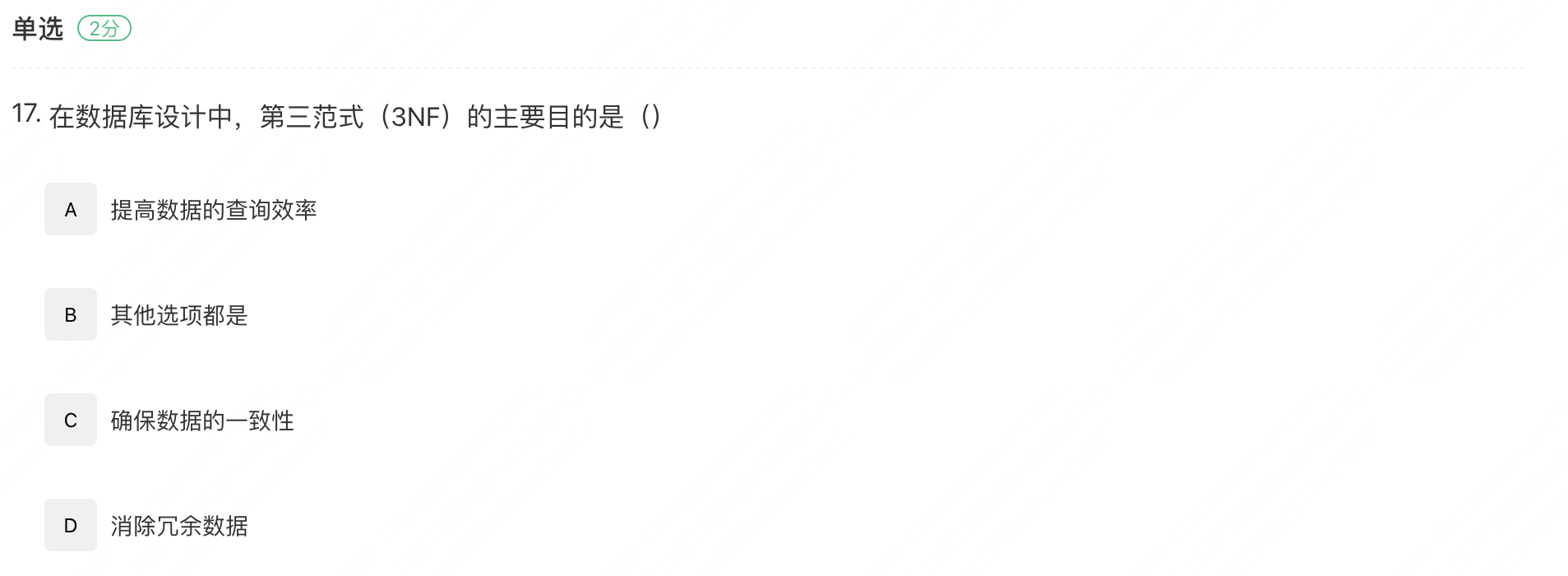
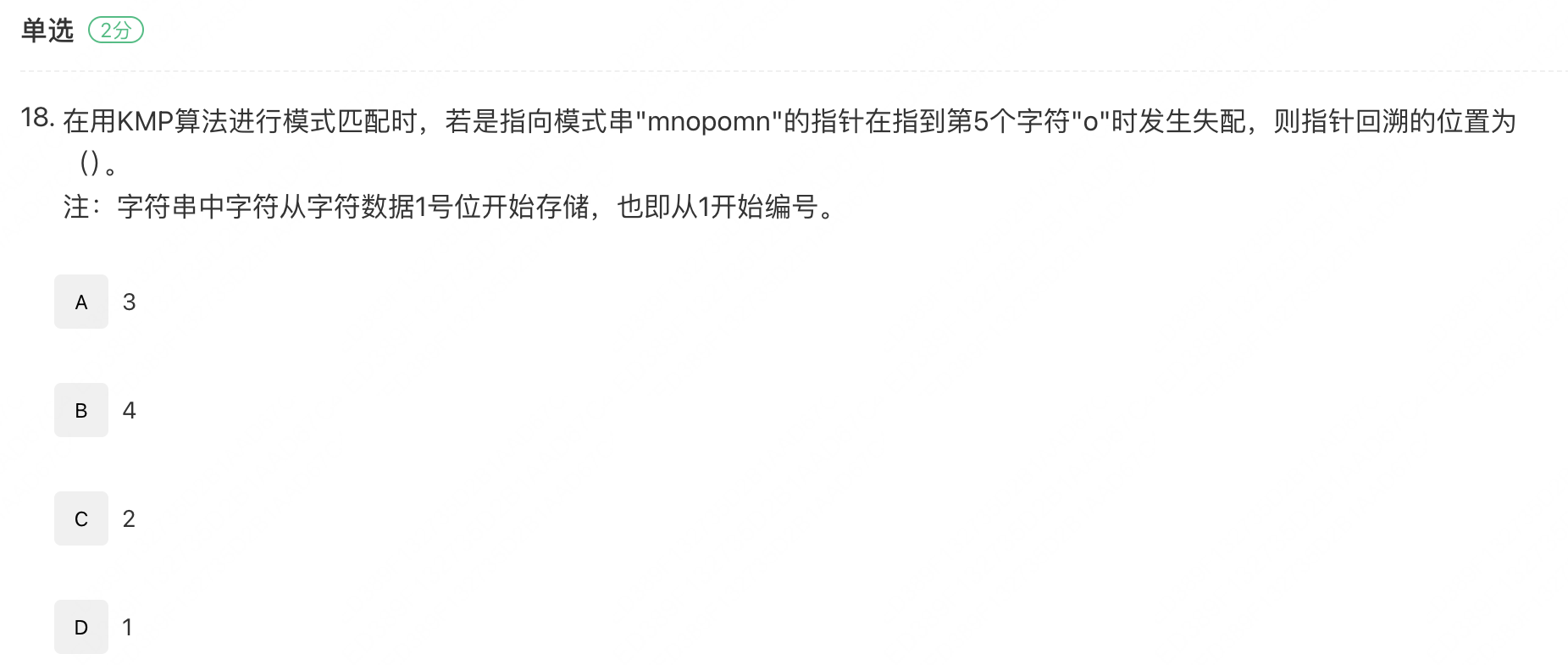
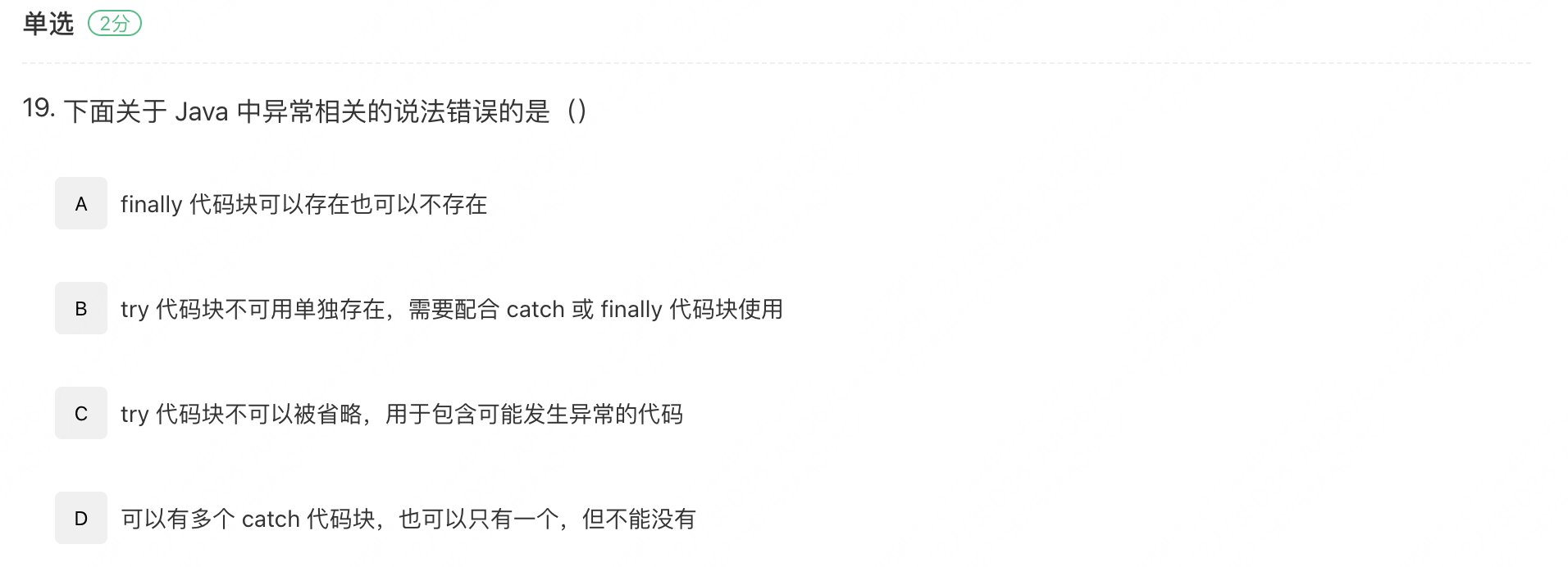
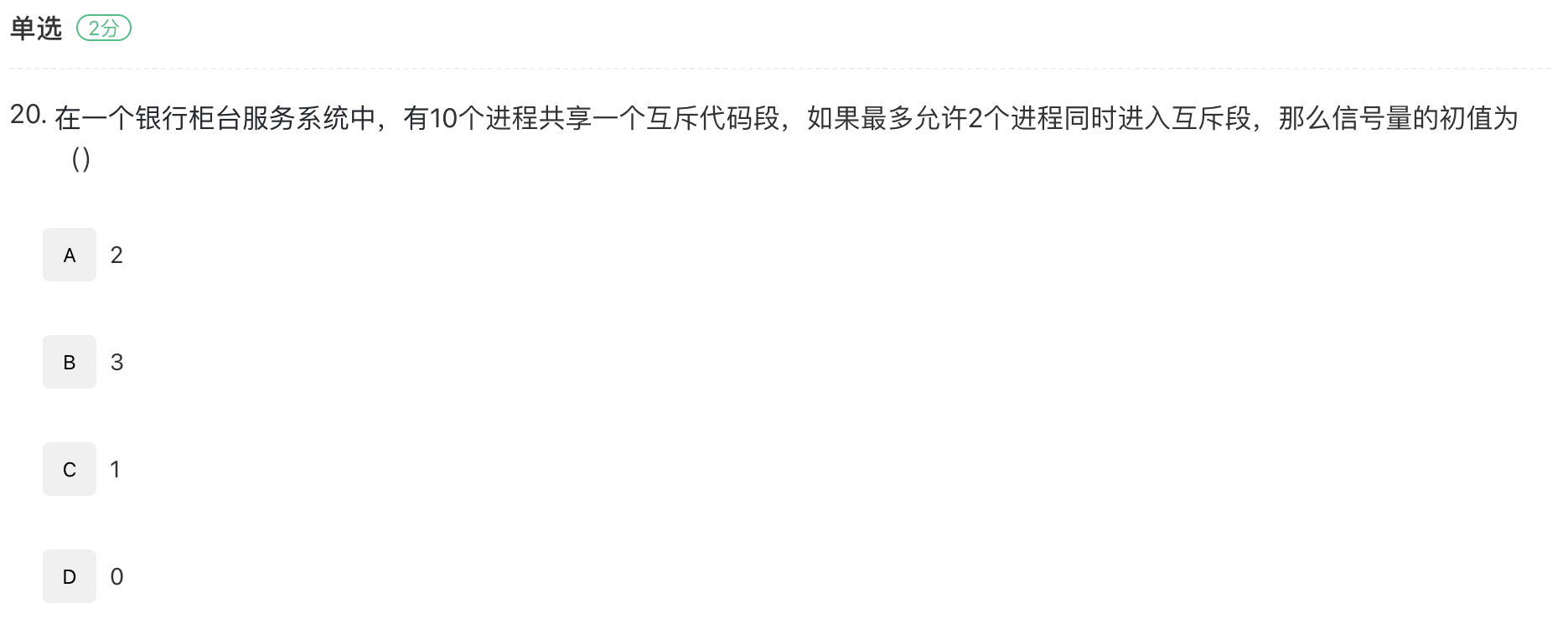
编程
1
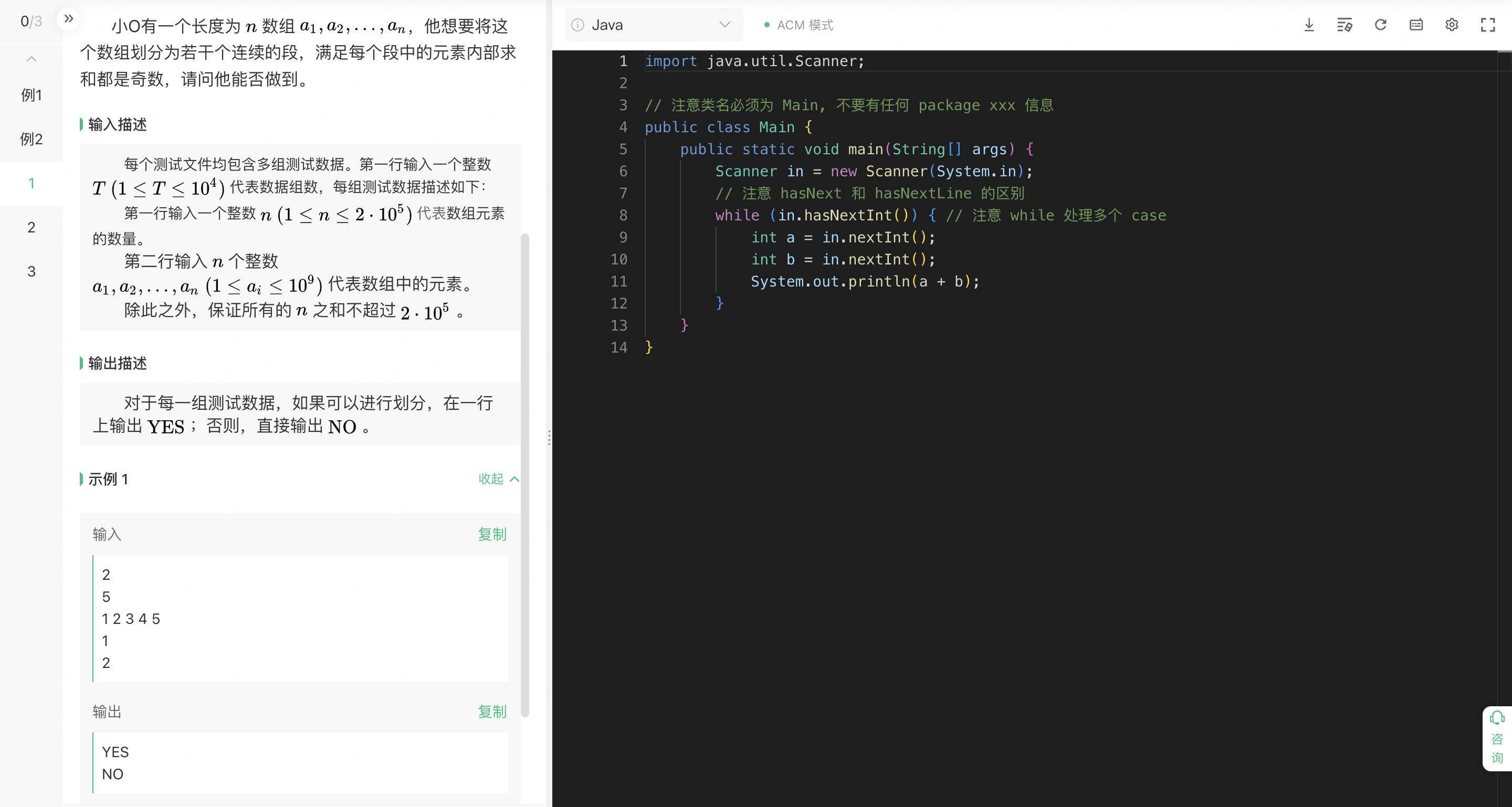
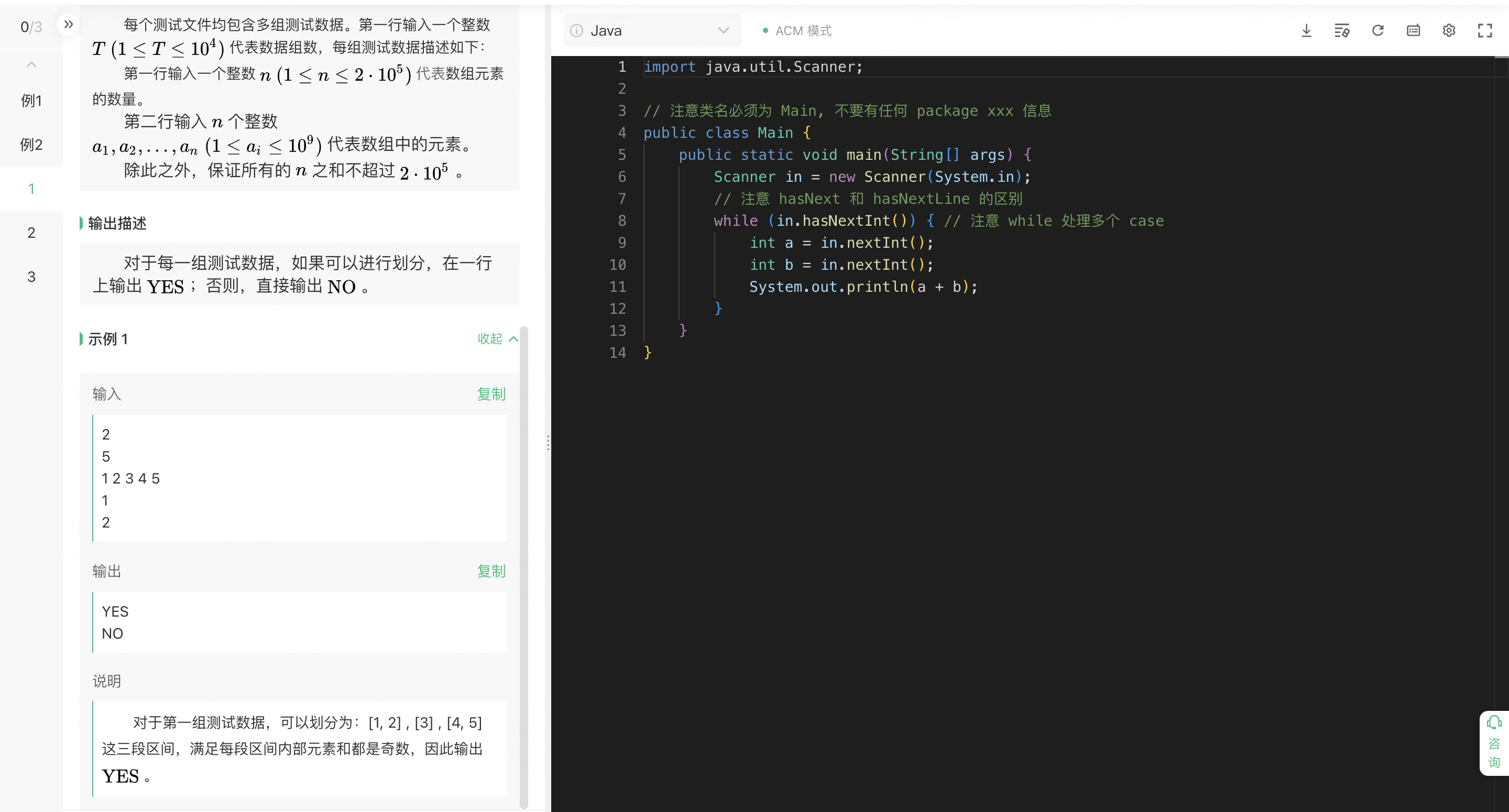
java
// Description: oppo-2025届秋招-移动端B卷-20240914-编程第1题
// Accept: 100%
// Date: 2024/09/14
package main;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
int T, n;
Scanner in = new Scanner(System.in);
while (in.hasNext()) {
T = in.nextInt();
in.nextLine();
while (T-- > 0) {
n = in.nextInt();
in.nextLine();
boolean yes = false;
for (int i = 0; i < n; i++) {
if (in.nextInt() % 2 == 1) {
yes = true;
}
}
System.out.println(yes ? "YES" : "NO");
}
}
}
}
2
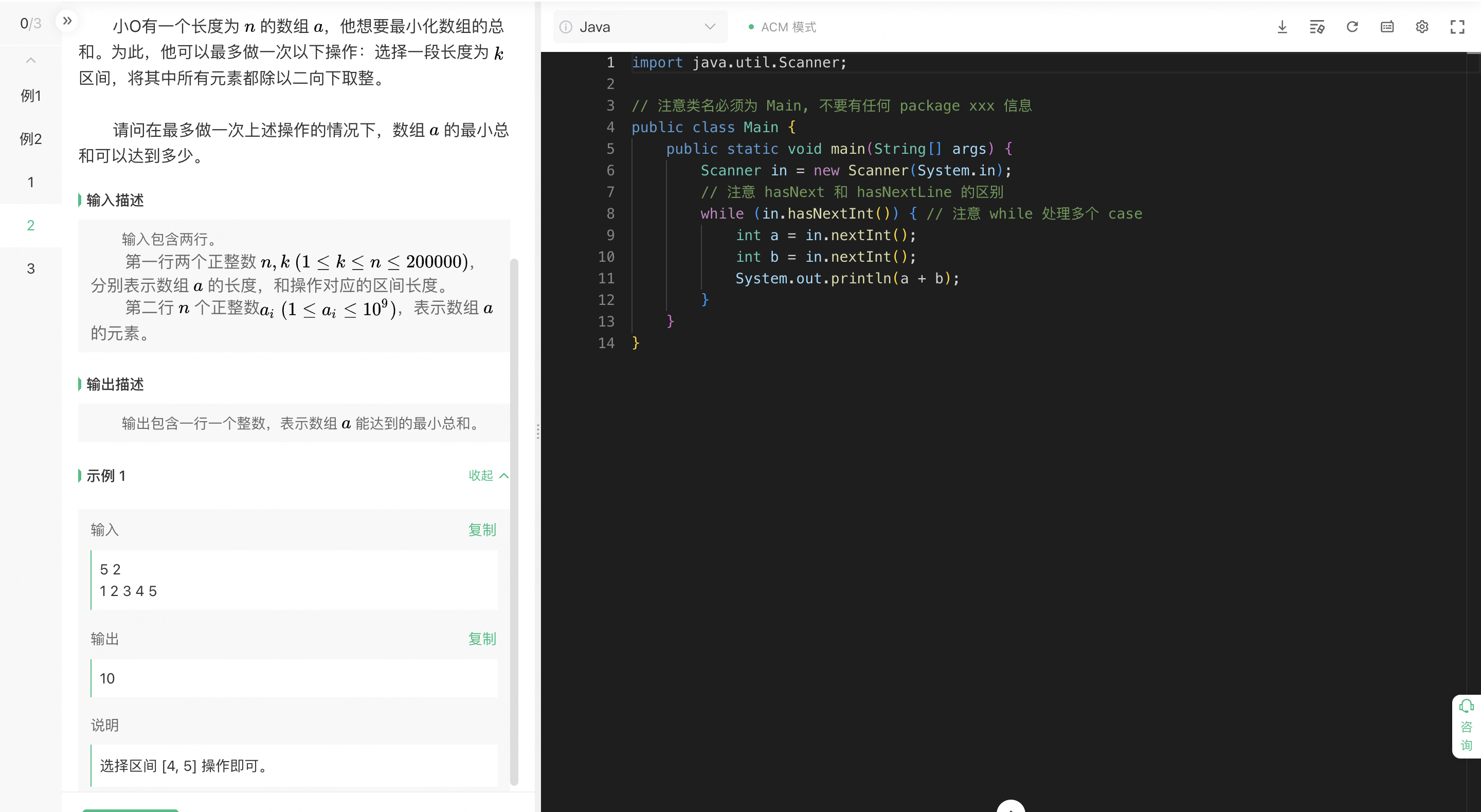
java
// Description: oppo-2025届秋招-移动端B卷-20240914-编程第2题
// Accept: 100%
// Date: 2024/09/14
package main;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
int n, k;
Scanner in = new Scanner(System.in);
while (in.hasNext()) {
n = in.nextInt();
k = in.nextInt();
long sum = 0, kmax, ksum, kodd_count_max;
int kodd_count = 0, kmax_index = 0;
int[] a = new int[n];
for (int i = 0; i < k; i++) {
a[i] = in.nextInt();
sum += a[i];
if (a[i] % 2 == 1) {
++kodd_count;
}
}
kmax = sum;
ksum = sum;
kodd_count_max = kodd_count;
for (int i = k; i < n; i++) {
a[i] = in.nextInt();
sum += a[i];
ksum += a[i] - a[i - k];
if (a[i - k] % 2 == 1) {
--kodd_count;
}
if (a[i] % 2 == 1) {
++kodd_count;
}
if (ksum > kmax) {
kmax = ksum;
kodd_count_max = kodd_count;
kmax_index = i - k + 1;
} else if (ksum == kmax) {
if (kodd_count > kodd_count_max) {
kodd_count_max = kodd_count;
kmax_index = i - k + 1;
}
}
}
for (int i = kmax_index; i < k + kmax_index; i++) {
sum -= (a[i] - (a[i] / 2));
}
System.out.println(sum);
}
}
}
3
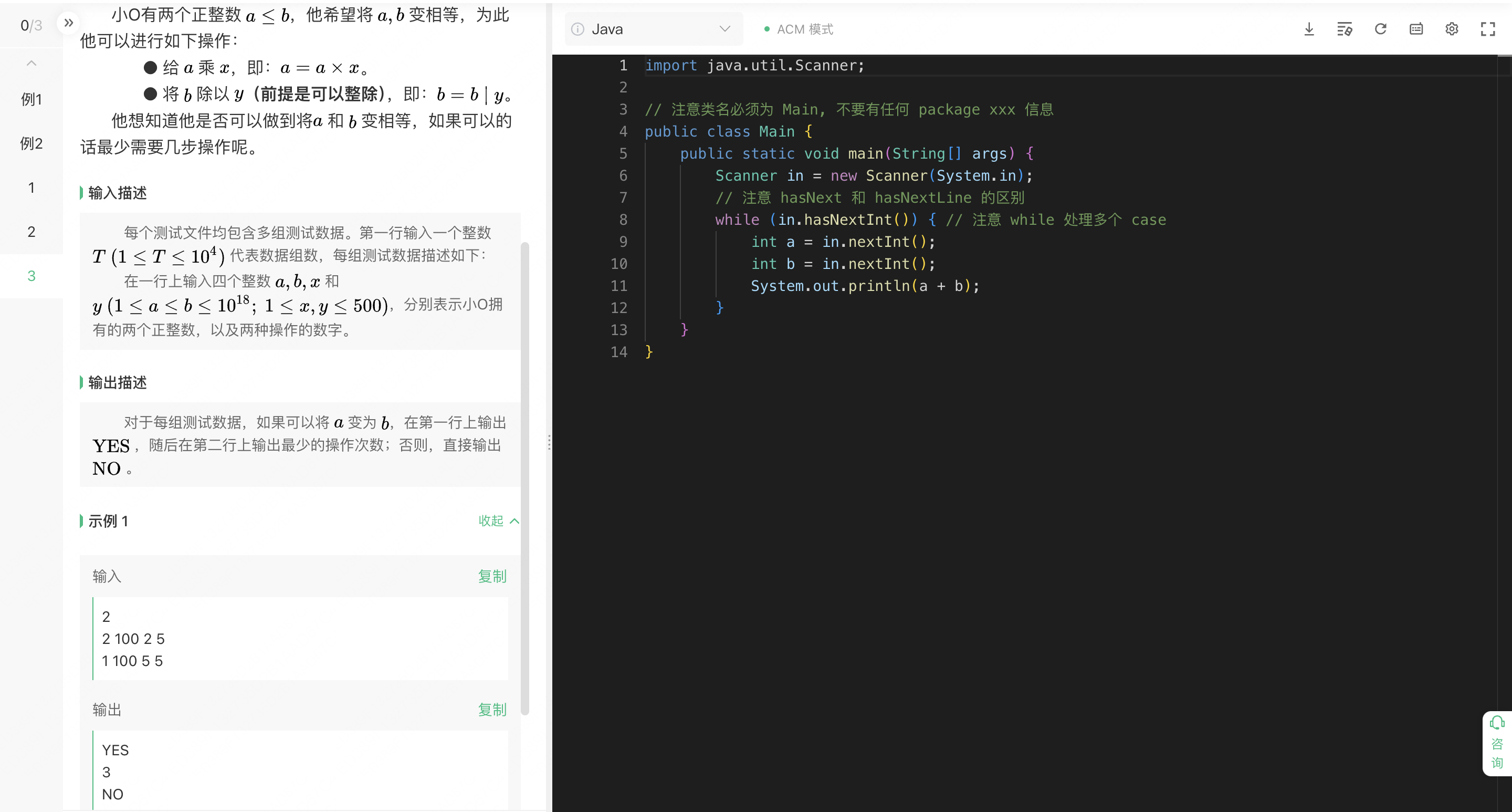
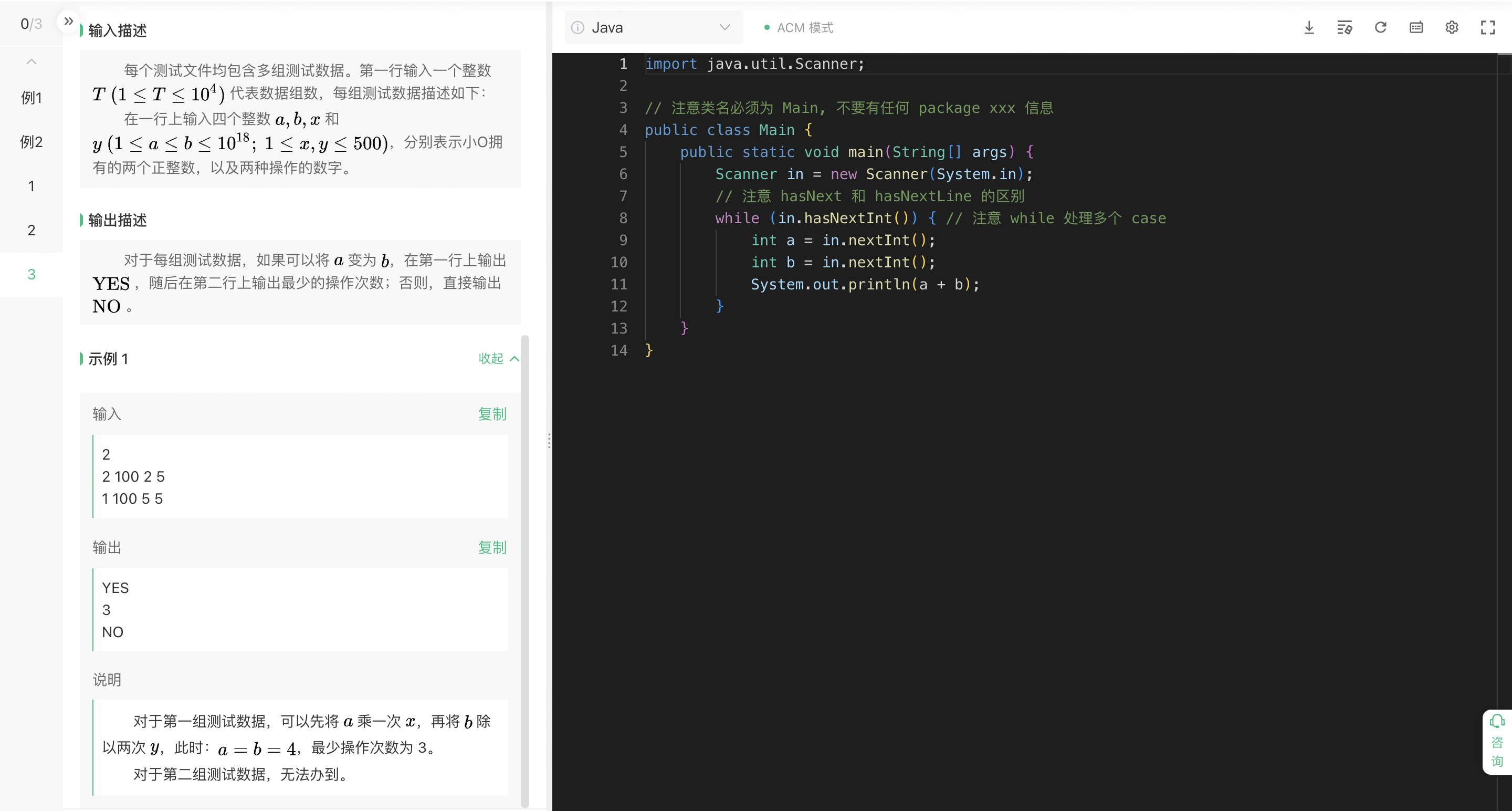
cpp
// Description: oppo-2025届秋招-移动端B卷-20240914-编程第3题
// Accept: 0%
// Date: 2024/09/14
#include <iostream>
#include <unordered_map>
#include <vector>
using namespace std;
class Node {
public:
unsigned long long v;
vector<Node *> children;
bool isA;
int depth = 0;
explicit Node(unsigned long long _v, bool _isA) : v(_v), isA(_isA) {}
void addChild(Node *n) {
n->depth = depth + 1;
children.emplace_back(n);
}
~Node() {
// delete rightChild;
}
};
int main() {
int T, x, y;
unsigned long long a_before, b_before, a, b;
cin >> T;
while (T-- > 0) {
int min = INT32_MAX;
cin >> a >> b >> x >> y;
a_before = a, b_before = b;
unordered_map<unsigned long long, Node *> aMap;
unordered_map<unsigned long long, Node *> bMap;
// 将a不断乘以x,并将结果节点存储图中,并指向此节点,直到a的值大于等于b
// 将b不断除以y,并将结果节点存储图中,并指向此节点,直到b的值小于等于a
Node *aRoot = new Node(a, true);
aMap[a] = aRoot;
Node *now = aRoot;
while (true) {
a = a * x;
if (a > b) {
break;
}
now->addChild(new Node(a, true));
now = now->children.at(0);
aMap[a] = now;
}
Node *bRoot = new Node(b, false);
bMap[b] = bRoot;
while (true) {
if (b % y != 0 || b < a_before) {
break;
}
b = b / y;
}
now = nullptr;
while (b <= b_before) {
Node *n = new Node(b, false);
if (aMap.find(b) != bMap.end() && aMap.find(b / x) != bMap.end()) {
aMap.find(b / x)->second->addChild(n);
}
if (now == nullptr) {
now = n;
} else {
now->addChild(n);
now = n;
}
if (n->v == b_before && !n->isA) {
if (n->depth < min) {
min = n->depth;
}
}
b = b * y;
}
if (min != INT32_MIN) {
cout << "YES" << endl;
cout << min << endl;
} else {
cout << "NO" << endl;
}
}
return 0;
}
/*
2
1 100 5 5
2 100 2 5
*/